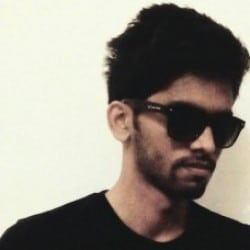
Anurag algoworks
IndividualForum Replies Created
-
Anurag
MemberOctober 4, 2018 at 2:31 pm in reply to: How to delete an index from Salesforce JSON Obj?Hi Chanchal,
You can remove an element from the JSONArray object using the remove() method. This method accepts an integer and removes the element in that particular index.
import org.json.JSONArray;
public class RemoveFromJsonArray {
public static void main(String args[]) throws Exception {
String [] myArray = {"JavaFX", "HBase", "JOGL", "WebGL"};
JSONArray jsArray = new JSONArray();
for (int i=0; i < myArray.length; i++) {
jsArray.put(myArray[i]);
}
System.out.println(jsArray);
jsArray.remove(3);
System.out.println("After deleting ::"+jsArray);
}
} -
Anurag
MemberOctober 3, 2018 at 2:10 pm in reply to: What are the different actions that can be made using Salesforce Data Loaders?Hi Anjali,
Using Data loaders we can perform 6 type of action.They are:
Insert – insertion of new records
Update – Updating existed records.
Upsert – Update and Insertion of records
Delete – Deletion of records.
Export – Extraction of all records.
Export All – It extracts all records from salesforce including Recycle Bin records. -
Anurag
MemberSeptember 27, 2018 at 2:05 pm in reply to: How many ways we can make field required in Salesforce?Hi Anjali,
Different ways to make field mandatory :
1. Make the field “Required” at the time of field creation by checking the “Required” check box.
2. Make the field Required through Page Layout by checking the “Required ” checkbook in Field Properties.
3. Validation Rules can also be used to make the field mandatory. In Error Condition Formula, one can use ISBLANK(“FieldName”);.
4. Triggers can be used to make field mandatory. Ex. If a user try to insert the record without the field which is required, we can throw the page massage specifying to fill up required fields.(Using Trigger.addError()).
5. One can make field mandatory through Visualforce.(If the field is getting referenced) by setting the required attribute in <apex:inoutField> to True. -
Anurag
MemberSeptember 26, 2018 at 2:42 pm in reply to: When do we use the data loader in Salesforce?Hi,
Use Data Loader when:
- You need to load 50,000 to 5,000,000 records. Data Loader is supported for loads of up to 5 million records. If you need to load more than 5 million records, we recommend you work with a Salesforce partner or visit the AppExchangefor a suitable partner product.
- You need to load into an object that is not yet supported by the import wizards.
- You want to schedule regular data loads, such as nightly imports.
- You want to export your data for backup purposes.
-
Anurag
MemberSeptember 25, 2018 at 1:57 pm in reply to: What is the Difference between the below two in Salesforce?Hi,
created and every time it is edited:
These simply means whenever we create or edit a record if criterion satisfies than workflow will fire.created and any time it is edited to subsequently meet criteria:
Here workflow fires only once,
1) Either when we create a record if criterion satisfies it will fire and if we update the same
record and again if criterion satisfies than it will not fire.2) Or When we create a record if criterion does not satisfies it will not fire and again if we update the same record if criterion satisfies it will fire.
-
Anurag
MemberSeptember 25, 2018 at 1:49 pm in reply to: What is the difference between managed packages and unmanaged package in Salesforce?Hi,
Managed Packages are the ones where the Code is Hidden and Can be easily Upgraded or PUshed with new updates to the code where as Unmanaged Packages have the Code Visible and can be edited by the Org in which it has been Installed. Unmanaged Packages cannot be Upgraded or Pushed - they are like one time coded.
-
Hi,
SaaS is beneficial to us in following ways:
- As SaaS is a subscription based, customers can always choose not to renew if they are dissatisfied
- Customers can avoid a large initial investment in an IT infrastructure and day to day hustle of maintaining infrastructure
- SaaS customer provides same provider infrastructure and also easy integration
- SaaS applications use a simple internet interface that makes easier for customer to use.
- SaaS always provide a latest platform to the customer with innovation.
-
Hi,
Use a matrix to display grouped data and summary information. You can group data by multiple fields or expressions in row and column groups. Matrices provide functionality similar to crosstabs and pivot tables. At run time, as the report data and data regions are combined, a matrix grows horizontally and vertically on the page. Values in matrix cells display aggregate values scoped to the intersection of the row and column groups to which the cell belongs. You can format the rows and columns to highlight the data you want to emphasize. You can also include drilldown toggles that initially hide detail data; the user can then click the toggles to display more or less detail as needed.
-
Anurag
MemberSeptember 20, 2018 at 6:50 am in reply to: Differentiate between Static SOQL and Dynamic SOQL in Salesforce.Hi Pavan,
Static SOQL is one which you write in square brackets. It is good to use when you didn't have any dynamic changes in the soql query.
For example:
list<Contact> con=[Select Id, LastName from Contact]
Dynamic SOQL refers to the creation of a SOQL string at runtime with Apex code. Dynamic SOQL enables you to create more flexible applications.To create dynamic query at runtime, we use Database.query() method.
For example,
String soql = 'SELECT id, name FROM Account';
List<Account> accList = Database.query(soql);
Thanks.
-
Anurag
MemberSeptember 19, 2018 at 2:01 pm in reply to: Define Javascript Remoting for Salesforce Apex Controllers.Hi Prachi,
Here’s a basic sample demonstrating how to use JavaScript remoting in your Visualforce pages
First, create an Apex controller called AccountRemoter:
global with sharing class AccountRemoter {
public String accountName { get; set; }
public static Account account { get; set; }
public AccountRemoter() { } // empty constructor@RemoteAction
global static Account getAccount(String accountName) {
account = [SELECT Id, Name, Phone, Type, NumberOfEmployees
FROM Account WHERE Name = :accountName];
return account;
}
}Other than the @RemoteAction annotation, this looks like any other controller definition.
To make use of this remote method, create a Visualforce page that looks like this:<apex:page controller="AccountRemoter">
<script type="text/javascript">
function getRemoteAccount() {
var accountName = document.getElementById('acctSearch').value;Visualforce.remoting.Manager.invokeAction(
'{!$RemoteAction.AccountRemoter.getAccount}',
accountName,
function(result, event){
if (event.status) {
// Get DOM IDs for HTML and Visualforce elements like this
document.getElementById('remoteAcctId').innerHTML = result.Id
document.getElementById(
"{!$Component.block.blockSection.secondItem.acctNumEmployees}"
).innerHTML = result.NumberOfEmployees;
} else if (event.type === 'exception') {
document.getElementById("responseErrors").innerHTML =
event.message + "<br/>\n<pre>" + event.where + "</pre>";
} else {
document.getElementById("responseErrors").innerHTML = event.message;
}
},
{escape: true}
);
}
</script><input id="acctSearch" type="text"/>
<button onclick="getRemoteAccount()">Get Account</button>
<div id="responseErrors"></div><apex:pageBlock id="block">
<apex:pageBlockSection id="blockSection" columns="2">
<apex:pageBlockSectionItem id="firstItem">
<span id="remoteAcctId"/>
</apex:pageBlockSectionItem>
<apex:pageBlockSectionItem id="secondItem">
<apex:outputText id="acctNumEmployees"/>
</apex:pageBlockSectionItem>
</apex:pageBlockSection>
</apex:pageBlock>
</apex:page> -
Anurag
MemberSeptember 19, 2018 at 1:53 pm in reply to: Describe a business process that can be automated using an approval process in Salesforce.Hi Anjali,
Business process automation (BPA) is the automation of business processes through technology, allowing businesses to cut costs and increase productivity.BPA is a means of automating recurring business processes through the use of software & different app integrations. Meaning, instead of having your employees to menial & simple tasks, you just let the software take care of it.
Approval Management – Let’s say you’re working in procurement & are ordering the new machinery. For the order to be completed, it has to go through the approval of 5 different general managers. Without automation, you’d have to hunt down each management member & ask for signatures. With approval management software, all you have to do is click “start the approval process.”
Employee Onboarding – Whatever your industry is, you’ve probably done employee onboarding before. The process is usually very structured (Fill in Document A, B, Email person C, etc.) – so rather than doing the whole thing from scratch, you can let workflow management software lead the entire process.
-
Anurag
MemberSeptember 19, 2018 at 1:50 pm in reply to: What Are The Three Types Of Bindings Used In Salesforce VisualForce? What Does Each Refer To?Hi Anjali,
There are three types of bindings used in Visualforce:
- Data binding: Data bindings refer to the data set in the controller.
- Action bindings: Action bindings refer to action methods in the controller.
- Component bindings: Component bindings refer to other Visualforce components.
-
Anurag
MemberSeptember 18, 2018 at 2:24 pm in reply to: What is Apex Managed Sharing in salesforce?Hi,
Salesforce allows you to control access to data at many different levels i.e. object-level, the record-level and the field-level. Here we will focus on methods for controlling access to data at the record-level. In the most of the scenario you can use Out-of-box sharing settings to grant record access, but there are few use cases where you have to write an Apex Managed Sharing to share the records with users or groups. Apex ManagedSharing allows you to use Apex Code to build sophisticated and dynamic sharing settings that aren’t otherwise possible. In this article I will show you how can use Flow and Process Builder to solve these requirements instead of using Apex code.
-
Anurag
MemberSeptember 18, 2018 at 1:13 pm in reply to: How to use remote site settings in salesforceHi Anjali,
Salesforce allows you to access external website resources from a Salesforce application for your organization. You can access these external websites through Visualforce pages, Apex Callout, and via XmlHttpRequest calls. To avoid accessing malicious websites from Salesforce.com, the website first needs to be registered with remote site settings. Once the site is registered, it can be used within Salesforce.com.
To register a new site, follow these steps:
1.Navigate to Setup | Administer | Security Controls | Remote Site Settings.
2.Click on the Remote Site Edit button to add a new site. -
Hi Anjali,
You can Automate business processes by building applications, known as flows, that collect, update, edit, and create Salesforce information. Then make those flows available to the right users or systems.Flows can either require user interaction—perhaps a wizard or guided UI for data entry—or run in the background on their own—perhaps something that automatically transfers records when a user’s role changes.The Cloud Flow Designer lets you design flows without writing any code.
-
Anurag
MemberSeptember 14, 2018 at 2:26 pm in reply to: What are some points to remember regarding Static Keyword in apex.Hi Prachi,
Static methods, variables, and initialization code have these characteristics.
They’re associated with a class.
They’re allowed only in outer classes.
They’re initialized only when a class is loaded.
They aren’t transmitted as part of the view state for a Visualforce page.In Apex, you can have static methods, variables, and initialization code. However, Apex classes can’t be static. You can also have instance methods, member variables, and initialization code, which have no modifier, and local variables.
-
Anurag
MemberSeptember 13, 2018 at 1:46 pm in reply to: What is the difference between “Export” and “Export All” in Data Loader in Salesforce?Hi Prachi,
Difference in both button functionality is very small:
When we use ‘Export’ button for any object in salesforce, all records( excluding records present in Recycle Bin) present in the system for that particular object are exported to a .csv file.
But when we use Export All, all records (including records present in Recycle Bin) for that particular object are exported to a .csv file. Deleted records present in recycle bin are also called ‘soft Deleted’ records.
-
Anurag
MemberSeptember 13, 2018 at 1:44 pm in reply to: When do you use a before vs. after trigger?Hi Avnish,
Generally we go for Before event if there is something which needs to be validated before the actual data is committed to database.
For example :
Let suppose you want to Insert new Account, but before the date is committed to database you want to validate if the Billing city is not null, if Null you will throw error and or else you will Insert record.And in case of After, once the record is inserted/ Updated or Deleted, based on some condition you want to do some process.
For example:
Once the record is Inserted you want to send mail to update some filed on object "XYZ". -
Anurag
MemberSeptember 13, 2018 at 1:39 pm in reply to: What do {!expressions} refer to when used in Visualforce components?Hi Prachi,
Anything inside the {! } delimiters is evaluated and dynamically replaced when the page is rendered or when the value is used.
-
Anurag
MemberSeptember 12, 2018 at 1:56 pm in reply to: What is community cloud model in Salesforce?Hi Chanchal,
Salesforce Community Cloud is an online social platform that enables companies to connect customers, partners, and employees with each other and the data and records they need to get work done. This next-generation portal combines the real-time collaboration of Chatter with the ability to share any file, data, or record anywhere and on any mobile device.
Community Cloud allows you to streamline key business processes and extend them across offices and departments, and outward to customers and partners. So everyone in your business ecosystem can service customers more effectively, close deals faster, and get work done in real time.
-
Hi Avnish,
Salesforce Analytics Cloud is a strong and secure cloud-based analytics program developed to help medium-sized businesses to large enterprises to implement rapid, iterative exploration of data, with results displayed via layers of dynamic visualization over underlying data sets. The presentation of information is highly optimized for mobile device users, with screens resized according to varying device types. All functionality comes at reasonable prices designed to meet the needs of diverse users, which is why we are certain both small and large companies will have their needs covered, regardless of the deployment method they’ve selected.
-
Anurag
MemberSeptember 11, 2018 at 2:05 pm in reply to: Can We Mass Delete Reports Using Apex (anonymous Apex)?Hi Anjali,
As of now, no such functionality exists in Salesforce.
-
Hi Chanchal,
Here are some of the tools:
- SoapUI
- Katalon Studio
- Postman
- Tricentis Tosca
- Apigee
- JMeter
- Rest-Assured
- Assertible
- Karate DSL
- No one-size-fit-all tools
-
Hi Chanchal,
Here is the List of 6 common Api Errors:
1. Using http:// instead of https://
2. Unexpected error codes
3. Using the wrong HTTP method
4. Sending invalid authorization credentials
5. Not specifying Content-Type or Accept header
6. APIs returning invalid content type when there is an error
-
Anurag
MemberSeptember 11, 2018 at 6:55 am in reply to: What is System.LimitException: Too many query rows: 50001 in Apex and Triggers?HI Prachi,
There is a limit to the number of records that that can be retrieved by the SOQL Query which is 50000 records. Attempting to query the records having the number more than this will give the error which you are getting.To query the records more than its limit, you need to use a Batch Class which provides a fresh limit of 50k records by resetting the limits.