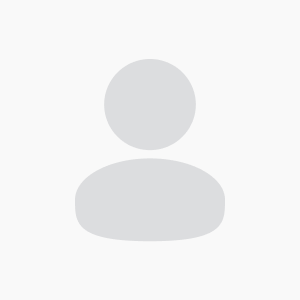
Laveena Agarwal
IndividualForum Replies Created
-
Laveena
MemberOctober 7, 2019 at 12:32 pm in reply to: Why Can't I Assign an Approval Step to a Queue for a Custom Object Approval Process in salesforce?Hi Deepak,
You can assign approvals on a detail object to queues, but only if you create a queue that can own the master object. This may not be possible if the master object cannot be owned by a queue.
These approval processes on the detail object that reference queues for the master object cannot be deployed via the metadata API, but can be created/modified through the standard UI. (you can deploy them with the assignment to a named user, and then switch it to the queue through the UI).
I've also heard of people working around the issue in the following way:
Creating the detail object without the master detail relationship
Creating queues for that object
Creating the approval process with the queues referenced
Adding the master detail relationship to the object.
You may want to try this, but it could be that it is no longer possible or unsupported by salesforce, it's certainly an ugly workaround.Thanks
-
Laveena
MemberOctober 4, 2019 at 7:17 am in reply to: Can we use fieldSet in the lightning component? if yes then how?Hi Piyush,
This component can be used to display child records or all the records of an object. See below component code.
<c:LightningTable
sObjectName="Opportunity"
fieldSetName="opportunitytable"
parentFieldName="AccountId"
parentRecordId="00190000016cYL6AAM"/>As you can see in the above component we have 4 attributes. These attributes can control the behaviour of the component. sObjectName : Name of the object which you want to query records from. (Required) fieldSetName: Name of the fieldset which will be used for the columns in the table. (Required) parentFieldName: If you want to display only child records of a parent then this should be specified. (Optional) parentRecordId: Parent record Id if you are displaying child records of a parent. (Optional) LightningTable is a parent component which is working as a table wrapper. This parent component is using a LightningCell component to display each cell of the row based on the data type. You can add more data types into the code as per your requirement. I am providing you all the code related to these components so you can update it as per your business use case. Fieldset is used to add/remove columns dynamically to the table.
<aura:component controller="LightningTableController" implements="flexipage:availableForAllPageTypes,flexipage:availableForRecordHome,force:hasRecordId,forceCommunity:availableForAllPageTypes,force:lightningQuickAction" access="global" >
<aura:attribute name="sObjectName" type="String"/>
<aura:attribute name="fieldSetName" type="String"/>
<aura:attribute name="fieldSetValues" type="List"/>
<aura:attribute name="tableRecords" type="List"/>
<aura:attribute name="parentFieldName" type="String"/>
<aura:attribute name="parentRecordId" type="String"/>
<aura:handler name="init" value="{!this}" action="{!c.doInit}"/>
<div class="slds">
<table class="slds-table slds-table--bordered">
<thead>
<tr>
<aura:iteration items="{!v.fieldSetValues}" var="field">
<th> {!field.label}</th>
</aura:iteration>
</tr>
</thead>
<tbody>
<aura:iteration items="{!v.tableRecords}" var="row">
<tr>
<aura:iteration items="{!v.fieldSetValues}" var="field">
<td>
<c:LightningCell record="{!row}" field="{!field}"/>
</td>
</aura:iteration>
</tr>
</aura:iteration>
</tbody>
</table>
</div>
</aura:component>LightningTableController [APEX Class]
public class LightningTableController {@AuraEnabled
public static String getFieldSet(String sObjectName, String fieldSetName) {
String result = '';
try{
SObjectType objToken = Schema.getGlobalDescribe().get(sObjectName);
Schema.DescribeSObjectResult d = objToken.getDescribe();
Map<String, Schema.FieldSet> FsMap = d.fieldSets.getMap();
system.debug('>>>>>>> FsMap >>> ' + FsMap);
if(FsMap.containsKey(fieldSetName))
for(Schema.FieldSetMember f : FsMap.get(fieldSetName).getFields()) {
if(result != ''){
result += ',';
}
String jsonPart = '{';
jsonPart += '"label":"' + f.getLabel() + '",';
jsonPart += '"required":"' + (f.getDBRequired() || f.getRequired()) + '",';
jsonPart += '"type":"' + (f.getType()) + '",';
jsonPart += '"name":"' + f.getFieldPath() + '"';
jsonPart += '}';
result += jsonPart;
}
}
catch(Exception e){
result += e.getLineNumber() + ' : ' + e.getMessage();
}
return '['+result+']';
}@AuraEnabled
public static String getRecords(String sObjectName, String parentFieldName, String parentRecordId, String fieldNameJson){List<sObject> lstResult = new List<sObject>();
String result = '[]';
try{
List<String> fieldNames = (List<String>) JSON.deserialize(fieldNameJson, List<String>.class);
Set<String> setFieldNames = new Set<String>();
String query = 'SELECT ' + String.join(fieldNames, ',') + ' FROM ' + sObjectName;
if(parentFieldName != NULL && parentFieldName != '' && parentRecordId != NULL){
query += ' WHERE ' + parentFieldName + '= '' + parentRecordId + ''';
}
for(sObject s : Database.query(query)){
lstResult.add(s);
}
if(lstResult.size() > 0) {
result = JSON.serialize(lstResult);
}
}
catch(Exception e){
result += e.getLineNumber() + ' : ' + e.getMessage();
}
return result;
}
}LightningTableController.js
({
doInit : function(component, event, helper) {
helper.doInit(component, event, helper);
}
})LightningTableHelper.js
({
doInit : function(component, event, helper) {
helper.getTableFieldSet(component, event, helper);
},getTableFieldSet : function(component, event, helper) {
var action = component.get("c.getFieldSet");
action.setParams({
sObjectName: component.get("v.sObjectName"),
fieldSetName: component.get("v.fieldSetName")
});action.setCallback(this, function(response) {
var fieldSetObj = JSON.parse(response.getReturnValue());
component.set("v.fieldSetValues", fieldSetObj);
//Call helper method to fetch the records
helper.getTableRows(component, event, helper);
})
$A.enqueueAction(action);
},getTableRows : function(component, event, helper){
var action = component.get("c.getRecords");
var fieldSetValues = component.get("v.fieldSetValues");
var setfieldNames = new Set();
for(var c=0, clang=fieldSetValues.length; c<clang; c++){ if(!setfieldNames.has(fieldSetValues[c].name)) { setfieldNames.add(fieldSetValues[c].name); if(fieldSetValues[c].type == 'REFERENCE') { if(fieldSetValues[c].name.indexOf('__c') == -1) { setfieldNames.add(fieldSetValues[c].name.substring(0, fieldSetValues[c].name.indexOf('Id')) + '.Name'); } else { setfieldNames.add(fieldSetValues[c].name.substring(0, fieldSetValues[c].name.indexOf('__c')) + '__r.Name'); } } } } var arrfieldNames = []; setfieldNames.forEach(v => arrfieldNames.push(v));
console.log(arrfieldNames);
action.setParams({
sObjectName: component.get("v.sObjectName"),
parentFieldName: component.get("v.parentFieldName"),
parentRecordId: component.get("v.parentRecordId"),
fieldNameJson: JSON.stringify(arrfieldNames)
});
action.setCallback(this, function(response) {
var list = JSON.parse(response.getReturnValue());
console.log(list);
component.set("v.tableRecords", list);
})
$A.enqueueAction(action);
},createTableRows : function(component, event, helper){
}
})Thanks
-
Hi Naresh,
System mode -
System mode is nothing but running apex code by ignoring user's permissions. For example, logged in user does not have create permission but he/she is able to create a record.
In system mode, Apex code has access to all objects and fields— object permissions, field-level security, sharing rules aren't applied for the current user. This is to ensure that code won’t fail to run because of hidden fields or objects for a user.
In Salesforce, all apex code run in system mode. It ignores user's permissions. Only exception is anonymous blocks like developer console and standard controllers. Even runAs() method doesn't enforce user permissions or field-level permissions, it only enforces record sharing.User mode -
User mode is nothing but running apex code by respecting user's permissions and sharing of records. For example, logged in user does not have create permission and so he/she is not able to create a record.
In Salesforce, only standard controllers and anonymous blocks like developer console run in user mode.Without Sharing keyword -
The 'without sharing' keyword is to ensure that the sharing rules (not permissions) for the current user are not enforced.
Example - Let's consider that the OWD for Account is private, no account records are owned by or shared with an user 'u' and a "without sharing" class called MyClass is fetching account records in a list. Now if class 'MyClass' is run by user 'u' then account records will be fetched. Remember that whether the user 'u' is having full CRUD or having no CRUD, records will be fetched.With Sharing keyword -
The with sharing keyword allows you to specify that the sharing rules (and not permissions) for the current user be taken into account for a class. You have to explicitly set this keyword for the class because Apex code runs in system mode.
Example - Let's consider that the OWD for Account is private, no account records are owned by or shared with an user 'u' and a "with sharing" class called MyClass is fetching account records in a list. Now if class 'MyClass' is run by user 'u' then no records will be fetched. Remember that whether the user 'u' is having full CRUD or having no CRUD record will not be fetched.Please look into the below links:
http://aluniya.blogspot.com/2014/02/salesforce-system-mode-user-mode-and.html
http://www.infallibletechie.com/2012/12/class-with-sharing-and-without-sharing.html
Thanks
-
Laveena
MemberSeptember 30, 2019 at 1:36 pm in reply to: What is the difference between Wire and Imperative function?Hi Saddam,
The functional programming paradigm was explicitly created to support a pure functional approach to problem solving. Functional programming is a form of declarative programming. In contrast, most mainstream languages, including object-oriented programming (OOP) languages such as C#, Visual Basic, C++, and Java, were designed to primarily support imperative (procedural) programming.
With an imperative approach, a developer writes code that describes in exacting detail the steps that the computer must take to accomplish the goal. This is sometimes referred to as algorithmic programming. In contrast, a functional approach involves composing the problem as a set of functions to be executed. You define carefully the input to each function, and what each function returns. The following table describes some of the general differences between these two approaches.
Thanks
-
Hi Nikita,
An avatar can be circular or a rounded rectangle, depending on usage. The default is a rounded rectangle and requires .slds-avatar as the base class.
Thanks
-
Laveena
MemberSeptember 30, 2019 at 1:32 pm in reply to: What are the draw back of Datatable in salesforce?Hi Nikita,
Disadvantages of Datatable in salesforce are-
It's not possible to have pick-list fields and lookup fields in Data Table.
Row Highlighting is not possible.
Anything which has got something to do with accessing the DOM is not possible.Thanks
-
Laveena
MemberSeptember 30, 2019 at 1:29 pm in reply to: what is the use of Accordion in lightning components?Hi Nikita,
An accordion allows a user to toggle (show and hide) the display of a section of content. In LightningFramework lightning:accordion component groups related content in a single container. Lightning:accordion can only used in a lightning componentif the version of component is 41.0 or later.
Thanks
-
Laveena
MemberSeptember 26, 2019 at 7:20 am in reply to: What is the use of Database.Stateful in batch Apex in Salesforce?Hi Hariom,
If you specify Database.Stateful in the class definition, you can maintain state across these transactions. When using Database.Stateful, only instance member variables retain their values between transactions. Static member variables don't retain their values and are reset between transactions.
Thanks
-
Laveena
MemberSeptember 25, 2019 at 1:35 pm in reply to: What are the different methods of force:recordData in Salesforce lightning component?Hi Nikita,
By using lightning data service, force:recordData tag is used to define the parameters for accessing, modifying, or creating a record.
Methods
Following methods are supported:1). getNewRecord : Set the record to target record.
2). reloadRecord : Perform the load function on init using current configuration value.
3). saveRecord : To save the record.
4). deleteRecord: To delete the record.
Thanks
-
Laveena
MemberSeptember 25, 2019 at 7:49 am in reply to: What is difference between Action support and Action function in Salesforce?Difference between both:
1. Action function can call the controller method from java script.
2. Action support adds AJAX support to another visualforce component and then call the controller method.
for example:<apex:outputpanel id="outptpnl">
<apex:outputText value="click here"/>
<apex:actionSupport event="onclick" action="{!controllerMethodName}" rerender="pgblck" />
</apex:outputpanel>Here action support adds AJAX to output panel, so once you click on output panel controller method will be called.
3. Action function cannot add AJAX support to another component. But from a particular component which has AJAX support(onclick, onblur etc) action function can be called to call the controller method.
Example:<apex:actionFunction name="myactionfun" action="{!actionFunMethod}" reRender="outptText"/>
<apex:inputcheckbox onclick="myactionfun" />In this example onlick of input checkbox "myactionfun" action function is called from where controller method "actionFunMethod" gets called.
Apart from this, the main difference between the "two" action support and action function is that, the action function can also be called from java script.
Example:<apex:actionFunction name="myactionfun" action="{!actionFunMethod}" reRender="outptText"/>
<apex:inputcheckbox onclick="myJavaMethod()" />
<script>
function myJavaMethod(){
myactionfun();// this call the action function
}
</script>Here onclick of the inputcheck box java script is called from where the action function gets called and ultimately your controller method.
-
Hi Piyush,
The testing process of Salesforce is same as any normal web-based application. The tester should have a clear perspective of the customizable features which are built during the test process. It helps them to focus on that cutomized code instead of the built-in Salesforce features.
Developer and tester should use the Sandbox environment (Test Enriovnment) for each of their purposes. Tested code in Sandbox environment is deployed to production from the Sandbox environment. It is assumed that the QA tester has the basic knowledge and understanding of the terms used in the Salesforce.
Thanks
-
Hi Saddam,
Single sign-on (SSO) is a session and user authentication service that permits a user to use one set of login credentials (e.g., name and password) to access multiple applications. SSO can be used by enterprises, smaller organizations, and individuals to mitigate the management of various usernames and passwords.
Thanks
-
Laveena
MemberSeptember 24, 2019 at 7:08 am in reply to: Can we Transfer Records from one org to another org in salesforce ? If Yes/No How ?Hi Piyush,
When we want to share information from one Salesforce org to another Salesforce org or getting records from another Salesforce Organization can be done in different methods, one of the method is Salesforce to Salesforce connection feature.
Salesforce to Salesforce:
Enable Salesforce to Slaesforce featureClick on Setup --> Build --> Customize --> Salesforce to Salesforce --> Settings
In the edit, click on enable and save.
Once it is enabled, we can't disable this setting, but we can control the information we want to share with the connections we make. We can stop sharing or modify sharing settings at any time.
After enabling, we get the information like email address, name, template names, etc. Whenever a connection is made first, we have to send invitation to another Organization, they receive the invitation from the email address, name and templates mentioned above.
We can change those address and template fields based on our requirement.
We have to check whether the salesforce to Salesforce connection has appropriate permissions like enabling manage connection permission on the profile of user.
Setting up a Connection:
Make sure to enable Salesforce to Salesforce in both sending and receiving Organizations. Now, we have to establish a relationship between the two organizations.Let us consider two Organizations org1 and org2. org1 is going to share records with org2, so the sender will be org1 and receiver will be org2.
Create an account and contact with an email address for org2 which will be receiving records, the contact will be used when invitation is sent.
In org1, connection's tab(if not appeared click on add in the tabs and add connection tab) click on new connection. Select a contact under the account which represents org2, click on save and send invitation. The invitation will be sent to the given mail address for the contact.
Org2 will receive invitation email with URL, upon clicking on that email it will redirect to org2 salesforce application. In the connection's tab, it will show connection detail like accept, decline and decide later. Upon accepting then the connection between two organizations is established.
Once the connection is established, next we can transfer records by publishing and subscribing objects.
In org1 click on publish in connection's tab and select the required objects to share, to select fields click on edit and select the fields of the object you want to share.
When objects and fields are published in org1, the receiving side(org2) doesn't receive automatically the data which is published. Org2 should click on subscribe then the published content appears.
Select the objects which you want to subscribe. There will be an option Auto-accept, if it is selected, then the records form publishing org are automatically accepted.
Map the required fields from the sending org1 to the fields available in receiving org2.
Note:
There are some considerations to field mapping like, only the same data type fields can be mapped.Thanks
-
Laveena
MemberSeptember 24, 2019 at 7:05 am in reply to: How to get the UserID of all the currently logged in users using Apex code in salesforce?Hi Prachi,
For apex Class, you may use and UserInfo.getUserId(), and for a Visualfroce page, you may use {!$User.Id}.
Thanks
-
Hi Saddam,
Canvas App Previewer is a development tool that lets you see what your canvas apps will look like before you publish them.
Thanks
-
Hi Saddam,
You can create update, or delete a large volume of records with the Bulk API, which is optimized for processing large sets of data. It makes it simple to load, update, or delete data from a few thousand to millions of records.
Thanks
-
Hi Saddam,
- Email logs should be available within 30 minutes of your request.
- Email logs are available for messages sent within the past 30 days before your request.
- Each email log can span a maximum of 7 days. To see email log data for a duration longer than seven days, create multiple requests.
- Email logs include emails sent through email actions, list email, and mass email, as long as the emails are sent through Salesforce.
Thanks
-
Hi Nikita,
Yes, Lightning is a Mobile View Controller.
Thanks
-
Laveena
MemberSeptember 20, 2019 at 12:40 pm in reply to: What is error code 30000 from REST API in Salesforce?Hi Yogesh,
To remove this error, just add maximum length of a field in Data Extension.
Thanks
-
Hi Saddam,
lightning:appHomeTemplate interface is used to enable your component to be used as a custom Lightning page template for pages of type App Page. This interface has no effect except when used within Lightning Experience and the Salesforce app.
Components that implement this interface appear in the Custom Templates section of the Lightning App Builder new page wizard for app pages.
Each template component should implement only one template interface. Template components shouldn’t implement any other type of interface, such as flexipage:availableForAllPageTypes or force:hasRecordId. A template component can’t multi-task as a regular Lightning component. It’s either a template, or it’s not.
Thanks
-
Laveena
MemberSeptember 20, 2019 at 12:33 pm in reply to: Why callouts in trigger are not possible in salesforce ?Hi Prachi,
The reason for it is that the apex trigger restricts database transaction until the callout is completed and the time limit for this is up to 120 seconds.
Thanks
-
Laveena
MemberSeptember 20, 2019 at 11:33 am in reply to: What is lightningsnapin:prechatUI in Salesforce?Hi Saddam,
This interface is used to indicate that a component can be used with Snap-Ins Pre-Chat.
This interface is a marker interface. A marker interface is a signal to the component’s container to add the interface’s behavior to the component. You don’t need to implement any specific methods or attributes in your component, you simply add the interface name to the component’s implements attribute.
In this case, the interface doesn’t add behavior but makes the custom component available as a pre-chat page from Snap-ins Setup. Without this interface, the component doesn’t appear as a pre-chat page option in Snap-ins Setup. Use this interface with the lightningsnapin:prechatAPI component to enable customization of your pre-chat user interface experience in Snap-ins for web.
This example shows the markup required to implement the lightningsnapin:prechatUI interface.
<aura:component implements="lightningsnapin:prechatUI">
<!-- ... -->
</aura:component>Thanks
-
Hi Saddam,
By Schema class, you can retrieve data from server without SOQL query.
for eg- get recordTypeId without soql query
Id contRecordTypeId = Schema.SObjectType.Contact.getRecordTypeInfosByName().get(‘NameOfRecordType’).getRecordTypeId();
Thanks
-
Laveena
MemberSeptember 19, 2019 at 12:13 pm in reply to: What is the use of future method in salesforce?Hi Nikita,
A future method runs in the background, asynchronously. You can call a future method for executing long-running operations, such as callouts to external Web services or any operation you'd like to run in its own thread, on its own time. Future , the method executes when Salesforce has available resources.
Thanks
-
Laveena
MemberSeptember 19, 2019 at 12:10 pm in reply to: What is the difference between JSON Parser and JSON Deserialization ?Hi Hariom,
JSON deserialization is a method used for JSON parsing in salesforce.
We can deserialize a JSON response into a class using the ‘JSON.deserialize’ method.
For more info you can go to:
https://developer.salesforce.com/docs/atlas.en-us.apexcode.meta/apexcode/apex_json_jsonparser.htm
Hope this helps
Thanks