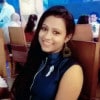
Nikita
IndividualForum Replies Created
-
Nikita
MemberAugust 20, 2019 at 6:36 am in reply to: How to convert Javascript objects into Salesforce JSON?You can use JSON.serialize() method .
for example
Account acc = new Account(Name = 'Account Name', Phone = '8888888888', Industry = 'Agriculture');
//Code to convert Account to JSON string
String str = JSON.serialize(acc);
system.debug('Account JSON Data - ' + str); -
Nikita
MemberAugust 20, 2019 at 6:28 am in reply to: How to embed multiple Salesforce Lightning Web Components using lightning layout?Hi Piyush,
You have to mention the size on the layout item. When you don't mention size on layout-item, it will become flexible in terms of width and each item occupy width according to its content. Total horizontal size is divided into 12 parts.
For Example
Size should be 6 for 50:50.
<div class="c-container"> <lightning-layout horizontal-align="spread" multiple-rows> <lightning-layout-item padding="around-small" size="6"> (My 1st LWC) </lightning-layout-item> <lightning-layout-item padding="around-small" size="6"> (My 2nd LWC) </lightning-layout-item> </lightning-layout> </div>
for more detail, you can refer https://lightningdesignsystem.com/utilities/grid/
-
Nikita
MemberAugust 20, 2019 at 6:16 am in reply to: How can I expose an Apex class as a REST WebService in Salesforce?Hi Laveena,
you can expose your Apex class and methods so that external applications can access your code and your application through the REST architecture. This is done by defining your Apex class with the @RestResource annotation to expose it as a REST resource. You can then use global classes and a WebService callback method.
Invoking a custom Apex REST Web service method always uses system context. Consequently, the current user’s credentials are not used, and any user who has access to these methods can use their full power, regardless of permissions, field-level security, or sharing rules.
Developers who expose methods using the Apex REST annotations should, therefore, take care that they are not inadvertently exposing any sensitive dataLook at the below piece of code for instance:-
global class AccountPlan { webservice String area; webservice String region; //Define an object in apex that is exposed in apex web service global class Plan { webservice String name; webservice Integer planNumber; webservice Date planningPeriod; webservice Id planId; } webservice static Plan createAccountPlan(Plan vPlan) { //A plan maps to the Account object in salesforce.com. //So need to map the Plan class object to Account standard object Account acct = new Account(); acct.Name = vPlan.name; acct.AccountNumber = String.valueOf(vPlan.planNumber); insert acct; vPlan.planId=acct.Id; return vPlan; } }
-
Nikita
MemberAugust 20, 2019 at 6:11 am in reply to: How can I create an account edit page using Lightning Component in Salesforce?Hi Laveena,
The simplest way to create a form that enables you to edit a record is to use the lightning:recordForm component. If you want to customize the form layout or preload custom values, use lightning:recordEditForm. If you want to customize a form more than the form-based components allow, use force:recordData.
you can refer https://developer.salesforce.com/docs/atlas.en-us.lightning.meta/lightning/data_service_save_record.htm
-
Nikita
MemberAugust 19, 2019 at 2:14 pm in reply to: What is the use of LDS in Salesforce Lightning Component?Hi Saddam,
LDS is tends for Lightning Data Service. Use Lightning Data Service to load, create, edit, or delete a record in your component without requiring Apex code. Lightning Data Service handles sharing rules and field-level security for you. In addition to simplifying access to Salesforce data, Lightning Data Service improves performance and user interface consistency.
-
Nikita
MemberAugust 19, 2019 at 2:11 pm in reply to: What is overlay Library in Salesforce Lightning Component?Hi Saddam,
Messages can be displayed in modals and popovers. Modals display a dialog in the foreground of the app, interrupting a user’s workflow and drawing attention to the message. Popovers display relevant information when you hover over a reference element.
Include one <lightning:overlayLibrary aura:id="overlayLib"/> tag in the component that triggers the messages, where aura:id is a unique local ID. Only one tag is needed for multiple messages.
You can also refer
-
lightning:combobox is an input element that enables single selection from a list of options. The result of the selection is displayed as the value of the input.
This component inherits styling from combobox in the Lightning Design System.
This example creates a list of options during init with a default selection.
<aura:component>
<aura:attribute name="statusOptions" type="List" default="[]"/>
<aura:handler name="init" value="{! this }" action="{! c.loadOptions }"/>
<lightning:combobox aura:id="selectItem" name="status" label="Status"
placeholder="Choose Status"
value="new"
onchange="{!c.handleOptionSelected}"
options="{!v.statusOptions}"/>
</aura:component> -
Nikita
MemberAugust 19, 2019 at 12:13 pm in reply to: Can we make a Lightning Component that shows in both the mobile and the desktop UI in salesforce ?yes, we can use Lightning Components directly in Lightning Experience, the Salesforce1 Mobile app, template-based communities, and custom standalone apps. Additionally, we can include Lightning components in a Visualforce page, that allowing us to use them in Salesforce Classic, Console, and Visualforce-based communities.
-
Nikita
MemberAugust 19, 2019 at 11:11 am in reply to: How to create a popup in Salesforce Lightning page?Hi Hariom,
you can easily get your answer please refer
-
Nikita
MemberAugust 19, 2019 at 8:28 am in reply to: Is there a limit for data.com records in Salesforce?Hi Achintya,
Yes, there are limits for Data.com Records. From Setup, in the Quick Find box, enter Users and then select Prospector Users. In the Data.com Users section, find your name and see what your monthly limit is and how many records you’ve already added or exported during the month. Your administrator sets the monthly allowance, which expires at the end of each month, regardless of whether you’ve met your limit.
-
Nikita
MemberAugust 19, 2019 at 6:59 am in reply to: Explain various dashboard components in Salesforce?Hi Piyush,
Dashboard Components are following types
Chart: Use a chart when you want to show data graphically. You can choose from a variety.
Gauge: Use a gauge when you have a single value that you want to show within a range of custom values.
Metric: Use a metric when you have one key value to display.
Table: Use a table to show a set of report data in column form.
Visualforce Page: Use a Visualforce page when you want to create a custom component or show information not available in another component type
Custom S-Control: Custom s-controls can contain any type of content that you can display in a browser, for example, a Java applet, an Active-X control, an Excel file, or a custom HTML Web form.
For more detail, you can refer
https://help.salesforce.com/articleView?id=dashboards_component_types.htm&type=5
-
Nikita
MemberAugust 19, 2019 at 6:29 am in reply to: What are the types of bindings used in Visualforce? What does each refer to?Hi Piyush,
There are three types of bindings used in Visualforce
- Data binding: Data bindings refer to the data set in the controller.
- Action bindings: Action bindings refer to action methods in the controller.
- Component bindings: Component bindings refer to other Visualforce components.
-
Hi Piyush,
Standard Controller:
- The standard controller in apex inherits all the standard object properties and standard button functionality directly.
- It contains the same functionality and logic that are used for standard Salesforce pages.
- It can be used with standard objects and custom objects.
- It can be extended to implement custom functionality using extensions keyword.
- It provides a save method to allow you to persist changes.
- You’d use this when you have a single object to manipulate.
Custom Controller:
- It is an Apex class that implements all of the logic for a page without leveraging a standard controller.
- Custom Controllers are associated with Visualforce pages through the controller attribute.
- Custom controller defines its own functionality.
- Use custom controllers when you want your Visualforce page to run entirely in system mode, which does not enforce the permissions and field-level security of the current user.
- You’d use this when your page isn’t dealing with a main object.
-
This reply was modified 5 years, 6 months ago by
Nikita.
-
Nikita
MemberAugust 14, 2019 at 7:41 am in reply to: How to retreive domain name from salesforce orgHi Deepak,
if you write a query select id, Domain from domain in the query editor, you will get the Id and Name of Your Domain.
-
This reply was modified 5 years, 6 months ago by
Nikita.
-
This reply was modified 5 years, 6 months ago by
-
Nikita
MemberAugust 14, 2019 at 6:33 am in reply to: What is the use of setStorable in Aura framework?Hi Saddam,
A storable action is a server action whose response is stored in the client cache so that subsequent requests for the same server method with the same set of arguments can be accessed from that cache. Server action is an Apex method that you invoke remotely from your Lightning Component.
To make an action storable, you simply call its setStorable() function.
-
Nikita
MemberAugust 14, 2019 at 4:41 am in reply to: What is the order of operation for triggers in Salesforce?Hi Piyush,
When you save a record with an insert, update, or upsert statement, Salesforce performs the following events in order.
- Loads the original record from the database or initializes the record for an upsert statement.
- Loads the new record field values from the request and overwrites the old values. If the request came from a standard UI edit page, Salesforce runs system validation to check the record for:
- Compliance with layout-specific rules
- Required values at the layout level and field-definition level
- Valid field formats
- Maximum field length
- When the request comes from other sources, such as an Apex application or a SOAP API call, Salesforce validates only the foreign keys. Before executing a trigger, Salesforce verifies that any custom foreign keys do not refer to the object itself. Salesforce runs user-defined validation rules if multiline items were created, such as quote line items and opportunity line items.
- Executes all before triggers.
- Runs most system validation steps again, such as verifying that all required fields have a non-null value, and runs any user-defined validation rules. The only system validation that Salesforce doesn't run a second time (when the request comes from a standard UI edit page) is the enforcement of layout-specific rules.
- Executes duplicate rules. If the duplicate rule identifies the record as a duplicate and uses the block action, the record is not saved and no further steps, such as after triggers and workflow rules, are taken.
- Saves the record to the database, but doesn't commit yet.
- Executes all after triggers.
- Executes assignment rules.
- Executes auto-response rules.
- Executes workflow rules.
- If there are workflow field updates, updates the record again.
- If the record was updated with workflow field updates, fires before update triggers and after update triggers one more time (and only one more time), in addition to standard validations. Custom validation rules, duplicate rules, and escalation rules are not run again.
- Executes processes and flows launched via processes and flow trigger workflow actions.When a process or flow executes a DML operation, the affected record goes through the save procedure.
- Executes escalation rules.
- Executes entitlement rules.
- If the record contains a roll-up summary field or is part of a cross-object workflow, performs calculations and updates the roll-up summary field in the parent record. Parent record goes through the save procedure.
- If the parent record is updated, and a grandparent record contains a roll-up summary field or is part of a cross-object workflow, performs calculations and updates the roll-up summary field in the grandparent record. Grandparent record goes through the save procedure.
- Executes Criteria Based Sharing evaluation.
- Commits all DML operations to the database.
- Executes post-commit logic, such as sending email.
-
Nikita
MemberAugust 14, 2019 at 4:33 am in reply to: What creative solutions have you come up with to navigate around a governor limit in Salesforce?Hi Piyush,
Governor Limits are limitations prevent certain functionality to be accomplished within SFDC.
-
Nikita
MemberAugust 14, 2019 at 4:23 am in reply to: Why can you not use Trigger.newMap in beforeInsert Salesforce trigger?Hi Piyush,
In before insert context your Trigger.NewMap will always be null because in before context records are not submitted to the database, so the Id is not generated yet. That's why in before insert we don't use Trigger.NewMap But in After insert, Id is generated so we can use Trigger.NewMap
-
Static resources allow you to upload content that you can reference in a Visualforce page, including archives (such as .zip and .jar files), images, style sheets, JavaScript, and other files.
Using a static resource is preferable to uploading a file to the Documents tab because:
- You can package a collection of related files into a directory hierarchy and upload that hierarchy as a .zip or .jar archive.
- You can reference a static resource in page markup by name using the $Resource global variable instead of hard-coding document IDs
- You can use relative paths in files in static resource archives to refer to other content within the archive. For example, in your CSS file, named styles.css, you have the following style:
You may also refer
https://help.salesforce.com/articleView?id=pages_static_resources.htm&type=5
-
Nikita
MemberAugust 13, 2019 at 12:26 pm in reply to: What is the use of <lightning-messages/> in sfdc?Hi Saddam,
You can refer
https://developer.salesforce.com/docs/component-library/bundle/ui:message/example
-
Hi Saddam,
Salesforce can create skinny tables to contain frequently used fields and to avoid joins. This can improve the performance of certain read-only operations. Skinny tables are kept in sync with their source tables when the source tables are modified.
Skinny tables can be created on custom objects, and on Account, Contact, Opportunity, Lead, and Case objects. They can enhance performance for reports, list views.
Skinny tables can contain the following types of fields.
Checkbox
Date
Date and time
Email
Number
Percent
Phone
Picklist (multi-select)
Text
Text area
Text area (long)
URL -
Nikita
MemberAugust 13, 2019 at 11:30 am in reply to: What is the Standard user field 'StartDay' in Salesforce?Hi Yogesh,
Both fields are not accessible through API. To get past this, you will need to create a custom formula field to pull in the data from the standard fields. You can then use the custom formula in your SOQL.
StartDay Formula field Data Type - Text Formula - TEXT(StartDay)
EndDay Formula field Data Type - Text Formula - TEXT(EndDay)
-
As we know, Apex runs in a multi-tenant environment, i.e., a single resource is shared by all the customers and organizations. So, it is necessary to make sure that no one monopolizes the resources and hence Salesforce.com has created the set of limits which governs and limits the code execution. Whenever any of the governor limits are crossed, it will throw an error and will halt the execution of the program.
From a Developer's perspective, it is important to ensure that our code should be scalable and should not hit the limits.
-
Nikita
MemberAugust 13, 2019 at 7:30 am in reply to: How can I embed a Visualflow in a Visualforce page in salesforce?Hi, Laveena
To add a flow to a Visualforce page, embed it by using the <flow: interview> component.
For more detail please refer
-
Nikita
MemberAugust 13, 2019 at 7:23 am in reply to: How many active assignment rules can I have in a lead/ case in Salesforce?Hi Laveena,
At a time only one assignment Rule can be active.