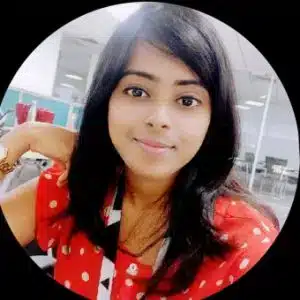
Parul
IndividualForum Replies Created
-
Parul
MemberSeptember 12, 2018 at 4:42 pm in reply to: How to query records from sObject for 50000+ records?Hi,
You can use Batchable class:
public class ContactBatchable implements Database.Batchable<sObject>, Database.Stateful {
Integer total = 0;public Database.QueryLocator start(Database.BatchableContext BC){
return Database.getQueryLocator('select Id from Contact');
}public void execute(
Database.BatchableContext BC,
List<sObject> scope){
total += scope.size();
}public void finish(Database.BatchableContext BC){
System.debug('total: ' + total);
}
}And then execute the following statement in developer console:
Database.executeBatch(new ContactBatchable(), 2000);
Thanks.
-
Parul
MemberSeptember 12, 2018 at 4:38 pm in reply to: How can I show a Visualforce page in the sidebar?Hi Shubham,
As Ajit and Nitish replied we can add page layout components and all. But if you are using Mozilla firefox, then you have to tell Firefox to accept third party cookies, because most of the page comes from the salesforce.com domain, and the iframe containing the VF page comes from force.com. Failing to allow third party cookies messes with the redirection.
Thanks.!!
-
Parul
MemberSeptember 12, 2018 at 4:30 pm in reply to: Can we get the site.com site url dynamically in class or vf page in Salesforce?Hello,
Try this once.. This has mine problem:
var prvsURL = document.referrer;
var getLoc = getParameterByName('loc',prvsURL);
function getParameterByName(name, url) {
name = name.replace(/[\[\]]/g, "\\$&");
var regex = new RegExp("[?&]" + name + "(=([^&#]*)|&|#|$)"),
results = regex.exec(url);
if (!results) return null;
if (!results[2]) return '';
return decodeURIComponent(results[2].replace(/\+/g, " "));
}
Hope this helps.
-
Parul
MemberSeptember 12, 2018 at 4:23 pm in reply to: What is the difference between returning null and returning ApexPages.currentPage()?Hello Himanshu,
The major difference is when you return null the constructors defined in the controller class don't execute.
While if you return whole page the page gets refreshed and constructors get executed only if you have made setRedirect flag set to true.Hence we have an option either to flushout the viewstate or not using this approach.Returning ApexPages.currentPage() will provide us ability to choose set redirect as true or false depending on the business need for the page.
If you are using reRender tags for ajax actions then we prefer null as to avoid whole refresh of page .
Thanks.!
-
Parul
MemberSeptember 12, 2018 at 4:19 pm in reply to: What is the best way to deploy profiles in Salesforce?Hello,
Use an appexchange application: Copado Deployer it will describe all the metadata on source org to produce a full XML of your profiles. it will apply a smart algorithm an remove any reference to non existing metadata on destination. Full Profile will be deployed successfully not leaving behind 1 permission.
Hope this helps.!!
-
Parul
MemberSeptember 12, 2018 at 4:08 pm in reply to: A trigger is running multiple times during a single save event in an org.How can this be prevented?Hi there,
In RecursiveTriggerHandler class, we need to have a static variable which is need to set to true by default.
public class RecursiveTriggerHandler{
public static Boolean isFirstTime = true;
}Find the sample trigger here:
trigger AccountTrigger on Account(after update){
Set<String> setOfAccId = new Set<String>();
if(RecursiveTriggerHandler.isFirstTime){
RecursiveTriggerHandler.isFirstTime = false;for(Account accObj : Trigger.New){
if(accObj .name != ‘Test Name’){
setOfAccId .add(accObj .accountId);
}
}// Use setOfAccId in your logic
}
}Once this trigger fired the static variable value will set to false and trigger will not fire again.
Hope this helps!
-
Parul
MemberSeptember 12, 2018 at 4:01 pm in reply to: When RollUp Summary field value changes, is any trigger is fire on Parent object?Hi Avanish,
If the record contains a roll-up summary field or is part of a cross-object workflow, performs calculations and updates the roll-up summary field in the parent record. Parent record goes through save procedure. So, updating the child inputs to a roll-up summary field can cause a trigger on an object to fire.
Consider the following example with a Master detail between Child and Parent and the following:
- Number field on Child called Num Field
- Roll-up summary on Parent that sums Num Field
Write the following trigger on Parent__c:
trigger ParentTrigger on Parent__c (before update) {
for (Parent__c p : Trigger.New) {
p.addError('Not going to save');
}
}Now go into the new or edit page for your Child object and fill in a value for Num Field and save the record and see what happens. You get:
Error: Invalid Data.
Review all error messages below to correct your data.
Not going to save
Next, blank out the Num Field and save and see what happens. No error is generated and the Childsaves.
So when the child record is saved and the field feeds into the roll-up summary the parent trigger is executed.
Thanks
-
Hi Avanish,
Salesforce Wave Analytics, also called Analytics Cloud, is a business intelligence (BI) platform from Salesforce.com that is optimized for mobile access and data visualization.
Wave Analytics is a service model in which elements of the data analytics process are provided through a public or private cloud. Cloud analytics applications and services are typically offered under a subscription-based or utility (pay-per-use) pricing model.
Hope this helps!
-
Parul
MemberSeptember 12, 2018 at 2:01 pm in reply to: Why is it necessary for most sales teams to use both Leads and Contacts?Hi
Once you convert the lead, the lead record is no longer accessible via the UI. So to access any of the personal contact information that was captured on lead records, sales team use the contact record.
Thanks.
-
Parul
MemberSeptember 12, 2018 at 1:59 pm in reply to: What is community cloud model in Salesforce?Hi
Community Cloud is a Salesforce platform that gives companies the tools to create branded online communities. These communities can be created for connecting with customers, external partners and employees.
Customer communities can be used for support and feedback. For companies with B2B business, partner communities can be used for qualifying leads, tracking revenue and providing product information and training. And internal employee communities can be resources for HR and IT information, as well as a place for discussion and collaboration.
Thanks.
-
Parul
MemberSeptember 12, 2018 at 1:54 pm in reply to: What is the scope of static variable in Apex?Hi,
I think the scope for a Static variable in Apex is the entire transaction. Any static variables will maintain their value across that one transaction.
Thanks.
-
Hi
Salesforce introduced Lightning Data Service in Winter 17 as a pilot program. Use Lightning Data Service to load, create, edit, or delete a record in your component without requiring Apex code. Lightning Data Service handles sharing rules and field-level security for you.
It’s built on highly efficient local storage that’s shared across all components that use it. Records loaded in Lightning Data Service are cached and shared across components. Components accessing the same record see significant performance improvements, because a record is loaded only once, no matter how many components are using it. Shared records also improve user interface consistency. When one component updates a record, the other components using it are notified, and in most cases, refresh automatically.
Advantages of Lightning Data Service
- No need to write any Apex class
- No need to write SOQL
- Field level security and record sharing is inbuilt
- CRUD operation supported
- Shared cache is used by all standard and custom components
- Auto notification to all components
- Supports offline in Salesforce 1
THanks.
-
Parul
MemberSeptember 12, 2018 at 1:39 pm in reply to: Difference between 'null' and 'undefined' In ApexHi
Undefined means a variable has been declared but has not yet been assigned a value, such as:
var TestVar;
alert(TestVar); //shows undefined
alert(typeof TestVar); //shows undefinednull is an assignment value. It can be assigned to a variable as a representation of no value:
var TestVar = null;
alert(TestVar); //shows null
alert(typeof TestVar); //shows objectTHanks
-
Parul
MemberSeptember 12, 2018 at 1:35 pm in reply to: how Lightning Data Service can improve Lightning Component performanceHi Anjali,
Lightning Data Service component, significantly reducing the complexity of your apps and pages. This reduced complexity means that you don’t have to spend as much time on performance and quality testing.
If there are many components on record detail page, then for each component there was SOQL to fetch data which affects the performance of the entire page. But if we use LDS it’s performance will improve as time lag to fetch data from server side will be saved.
Hope this will help you!
Thanks
-
This reply was modified 6 years, 2 months ago by
Parul.
-
This reply was modified 6 years, 2 months ago by
-
Parul
MemberSeptember 12, 2018 at 1:17 pm in reply to: What is the maximum size of the pdf generated on Salesforce Visualforce Attribute RenderAs?Hi
The maximum response size when creating a PDF file must be less than 15 MBbefore being rendered as a PDF file. This limit is the standard limit for all Visualforce requests.
Thanks.
-
Parul
MemberSeptember 12, 2018 at 9:15 am in reply to: What are the pros and cons when using a workflow rule field update vs. a formula field?Formula Field is a field that updated everytime you load the page, it's not writable and you can't fire a trigger or a workflow based on the change of a formula field, can't answer that since I don't know what are you trying to achieve.
Pros: can update a field to another field, can update more field types than available for formula fields. In general more robust then formula fields.
cons: subject to validation rules so could error out. Can't update a formula field
vs. a Formula Field?
Pros; automatically calculate a value based on different rules/criteria.
cons: Read-only, formula is static Can't use on certain fields like Rich Text Fields
Thanks
-
Parul
MemberSeptember 12, 2018 at 9:09 am in reply to: When should an extension be used instead of a custom controller?Hi
Extension Controller is an Apex class that extends the functionality of a standard or custom controller.
It helps us to leverage the built-in functionality of a standard controller but override one or more actions, such as edit, view, save, or delete.
We can create new actions.
If used with standard controller it respects user permissions. I.e. if used with standard controller, the logic from the controller extension does not execute in system mode. Instead, it executes in user mode, in which permissions, field-level security, and sharing rules of the current user applyExample:
Extension controller name : StdConExtensionCon
Thanks.
-
Parul
MemberSeptember 12, 2018 at 6:57 am in reply to: Where can we use List custom setting and hierarchy custom setting in Salesforce?Custom setting like as Custom object where you can store your data .
major difference is :
1 – Do not need to Query to access Data We can directly access them using methods
2 –
List custom setting : Every User can Access
hierarchy custom setting : restrict data by user by Using ( User Id) .3 – No owner is assigned when a custom setting is created
Thanks
-
Parul
MemberSeptember 12, 2018 at 5:11 am in reply to: What is the use of pagination in Visualforce?Hi
Pagination is the process of taking a complete document or a large number of records and breaking that document/large number of records into separate pages for viewing within the Salesforce. So when we need to break that document/large number of records into separate pages for viewing then use Pagination
Thanks
-
Parul
MemberSeptember 12, 2018 at 5:07 am in reply to: Why do we use extensions while creating the Salesforce Visualforce Page?Hi
A controller extension is an Apex class that extends the functionality of a standard or custom controller. Use controller extensions when:You want to leverage the built-in functionality of a standard controller but override one or more actions, such as edit, view, save, or delete.
Thanks
-
Parul
MemberSeptember 12, 2018 at 5:04 am in reply to: What is the lifecycle of an application in Salesforce?Application Lifecycle is the process of managing an app’s development, from design to final release, and establishing a framework for managing changes. The typical application lifecycle starts with the design of a new app or feature. The app is planned based on requirements analysis and specifications. Next, the app is implemented per the specifications and then tested. The new app is staged for final testing before it gets deployed to production. This cycle repeats for every new app or feature. It’s also used for app maintenance, such as when features are enhanced or bugs are fixed.
Thanks
-
Parul
MemberSeptember 12, 2018 at 5:02 am in reply to: How can I increase the Standard Date field year Range in Visualforce?Hi
$j(document).ready(function() {
var startYear=1985;
var endYear=2024;
var htmlStr='';
if(startYear<endYear){
for(i=startYear;i<endYear+1;i++){
htmlStr += "<option value=""+i+"">"+i+"</option>";
}
$j('#calYearPicker').html(htmlStr);
}
$j('#sidebarDiv #hideThisHomePageComp').parent().parent().hide();
}
);
</script>Thanks
-
Hi Prachi
We need to implement the following “force: lightningQuickAction” so that we can use the component as a Quick Action.
Hope this will help you!
-
Parul
MemberSeptember 12, 2018 at 4:55 am in reply to: Can We Mass Delete Reports Using Apex (anonymous Apex)?Hi
Salesforce has not exposed any API for Reports so We Mass Delete Reports by moving all reports to in new folder and then import your reports folder in Eclipse including all reports to be deleted and then delete the reports folder in eclipse. It will delete all the reports at once.
Thanks
-
Parul
MemberSeptember 12, 2018 at 4:51 am in reply to: Why can we not query fields of type long text in Salesforce?Hi
You can query records for long text type field but you can’t get these fields in WHERE clause because the fields to be used in where clause if their length is greater than 255 which give incorrect result.
Thanks