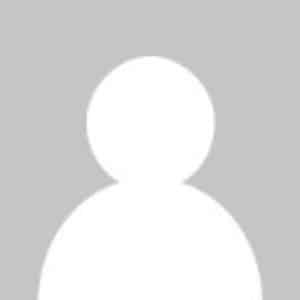
Saddam Hussain
IndividualForum Replies Created
-
Saddam
MemberJanuary 23, 2020 at 10:21 am in reply to: How to use Lightning Component to update records on serveryou can use aura:id in component and can get value using component.find('nameOfAuraId').get('v.value')
-
Hi,
A PageReference is a reference to an instantiation of a page. Among other attributes, PageReferences consist of a URL and a set of query parameter names and values.
Use a PageReference object:
· To view or set query string parameters and values for a page
· To navigate the user to a different page as the result of an action methodMethods
PageReference methods are all called by and operate on a particular instance of a PageReferenceThe table below describes the instance methods for PageReference.
getAnchor()
Returns the name of the anchor referenced in the page’s URL. That is, the part of the URL after the hashtag (#).
getContent()
Returns the output of the page, as displayed to a user in a Web browser.
getContentAsPDF()
Returns the page as a PDF, regardless of the <apex:page> component's renderAs attribute.
getCookies()
Returns a map of cookie names and cookie objects, where the key is a String of the cookie name and the value contains the list of cookie objects with that name.
getHeaders()
Returns a map of the request headers, where the key string contains the name of the header, and the value string contains the value of the header.
getParameters()
Returns a map of the query string parameters that are included in the page URL. The key string contains the name of the parameter, while the value string contains the value of the parameter.
getRedirect()
Returns the current value of the PageReference object's redirect attribute.
getUrl()
Returns the relative URL associated with the PageReference when it was originally defined, including any query string parameters and anchors.
setAnchor(String)
Sets the URL’s anchor reference to the specified string.
setCookies(Cookie[])
Creates a list of cookie objects. Used in conjunction with the Cookie class.
setRedirect(Boolean)
Sets the value of the PageReference object's redirect attribute. If set to true, a redirect is performed through a client side redirect. -
Saddam
MemberOctober 21, 2019 at 12:56 pm in reply to: How to bypass validation rules without adding anything in validation rule ?Hi,
Here is one strategy to bypass this behavior:
Add a "Skip Validation" Checkbox field to the object
Set the "Skip Validation" field to TRUE in a before trigger.
Add logic to your validation rules so that they do not execute if "Skip Validation" is set to TRUE. -
Saddam
MemberOctober 21, 2019 at 9:58 am in reply to: how to show toast message box in a lightning component ?Hi,
A toast displays a message below the header at the top of a view. The message is specified by the message attribute.
force:showToast is not available on login pages.
This example displays a toast message "Success! The record has been updated successfully.
showToast : function(component, event, helper) {
var toastEvent = $A.get("e.force:showToast");
toastEvent.setParams({
"title": "Success!",
"message": "The record has been updated successfully."
});
toastEvent.fire();
} -
Saddam
MemberOctober 18, 2019 at 1:20 pm in reply to: Why toast message is not displayed when the component is shown in a application?Hi,
This event is handled by the one.app container. It’s supported in Lightning Experience, Salesforce1, and Lightning communities.
-
Saddam
MemberOctober 18, 2019 at 1:18 pm in reply to: How to implement pagination in lightning component?Hi,
you can check here http://sfdcmonkey.com/2017/01/26/display-record-with-pager-buttons-lightning-component/
-
Saddam
MemberOctober 18, 2019 at 1:16 pm in reply to: What are the ways leads can be captured with Salesforce?Hi,
1. You can create Lead from UI
2. Also from email to lead
3. Web to lead
4. Customization (Integration point depending upon business rule)
5. Using Data Load -
Saddam
MemberOctober 17, 2019 at 7:28 am in reply to: How can I call a mock class in test class in salesforce?Hi,
Testing HTTP Callouts by Implementing the HttpCalloutMock Interface
Provide an implementation for the HttpCalloutMock interface to specify the response sent in the respond method, which the Apex runtime calls to send a response for a callout.global class YourHttpCalloutMockImpl implements HttpCalloutMock {
global HTTPResponse respond(HTTPRequest req) {
// Create a fake response.
// Set response values, and
// return response.
}
}Now that you have specified the values of the fake response, instruct the Apex runtime to send this fake response by calling Test.setMock in your test method. For the first argument, pass HttpCalloutMock.class, and for the second argument, pass a new instance of your interface implementation of HttpCalloutMock, as follows:
Test.setMock(HttpCalloutMock.class, new YourHttpCalloutMockImpl());
-
Saddam
MemberOctober 17, 2019 at 7:25 am in reply to: How can I create an edit button in lightning component in salesforce?Hi,
You can take advantage of built-in events to display modals that let you create or edit records via an Aura component.
The force:createRecord and force:editRecord events display a create record page and edit record page in a modal based on the default custom layout type for that object.The following example contains a button that calls a client-side controller to display the edit record page. Add this example component to a record page to inherit the record ID via the force:hasRecordId interface.
<aura:component implements="flexipage:availableForRecordHome,force:hasRecordId" >
<aura:attribute name="recordId" type="String" />
<lightning:button label="Edit Record" onclick="{!c.edit}"/>
</aura:component><aura:component implements="flexipage:availableForRecordHome,force:hasRecordId" >
<aura:attribute name="recordId" type="String" />
<lightning:button label="Edit Record" onclick="{!c.edit}"/>
</aura:component> -
Saddam
MemberOctober 17, 2019 at 7:21 am in reply to: How to use a custom setting in apex class in salesforce?Hi,
Custom settings are similar to custom objects and enable application developers to create custom sets of data, as well as create and associate custom data for an organization, profile, or specific user. All custom settings data is exposed in the application cache, which enables efficient access without the cost of repeated queries to the database. This data can then be used by formula fields, validation rules, flows, Apex, and the SOAP API.
There are two types of custom settings:
List Custom Settings
A type of custom setting that provides a reusable set of static data that can be accessed across your organization. If you use a particular set of data frequently within your application, putting that data in a list custom setting streamlines access to it. Data in list settings does not vary with profile or user, but is available organization-wide. Examples of list data include two-letter state abbreviations, international dialing prefixes, and catalog numbers for products. Because the data is cached, access is low-cost and efficient: you don't have to use SOQL queries that count against your governor limits.Hierarchy Custom Settings
A type of custom setting that uses a built-in hierarchical logic that lets you “personalize” settings for specific profiles or users. The hierarchy logic checks the organization, profile, and user settings for the current user and returns the most specific, or “lowest,” value. In the hierarchy, settings for an organization are overridden by profile settings, which, in turn, are overridden by user settings.Custom Setting Examples
The following example uses a list custom setting called Games. Games has a field called GameType. This example determines if the value of the first data set is equal to the string PC.List<Games__C> mcs = Games__c.getall().values();
boolean textField = null;
if (mcs[0].GameType__c == 'PC') {
textField = true;
}
system.assertEquals(textField, true); -
Saddam
MemberOctober 16, 2019 at 1:56 pm in reply to: What is the difference between Streaming API PushTopic,Generic Streaming and Platform events ?Hi,
Very generally, the Streaming API was created primarily to update UI components(Source) where as Platform Events are for integrations via a Message Bus.
With a PushTopic from the Streaming API you are limited to receiving events notifications when the configured query and event occur. There is no capacity to manually generate these events beyond causing the criteria required by the query and event.
Generic Streaming via the Streaming API is similar to Push Topics but is not bound to specific changes to Salesforce data. Instead you control when to publish an event. The trade off is that the payload of the event is a 3000 character string rather than a defined structure like that of a PushTopic.
Platform Events provide the defined notification structure of a PushTopic with a more flexible model than Generic Streaming for raising and subscribing to the events. They can be published and subscribed to via Apex in addition to the APIs.
-
Hi,
Core Features of Salesforce CRM
Contact Management
Customer Engagement Tools
Workflow Creation
Task Management
Opportunity Tracking
Collaboration Tools
Analytics
Intuitive, Mobile-Ready DashboardFeatures for Marketers
Marketing Leads Monitoring
Social Media Integration
Email IntegrationFeatures for Salespeople
Sales Leads Monitoring
Communities for Sales
Sales Forecasting -
Saddam
MemberOctober 16, 2019 at 1:50 pm in reply to: What feature in Salesforce is needed to restrict access to that object?Hi,
Sharing rule is nothing but extending the permissions given in the Owd criteria for a bunch of users. You can assign this to roles,single user,or a group of users. Sharing rule will extend access in one direction means
1) If u give OWD as private< you can change it to read , read/write , read/write/transfer.
2)If u give OWD as read< you can change it to read/write , read/write/transfer.
3)If u give OWD as read/write< you can change it to read/write/transfer.
4)If u give OWD as read/write/transfer.< you cannot change. -
Hi,
you can use this to handle event in visualforce page
$A.eventService.addHandler({ "event": "c:LookupEvent", "handler" : visualForceFunction});
-
Saddam
MemberOctober 15, 2019 at 11:49 am in reply to: How can we add watermark in visualforce pdf?Hi,
<img src="{!URLFOR($Resource.tran_watermark)}" width="100%" height="100%" style="position:fixed; background-repeat:repeat; left:0cm; top:0cm; z-index:0; display:{!IF(quote.status == 'approved', 'none', '')}"/>
go through the following link which gives a sample code you can test in your org to create a watermark on PDF generated by a VF :
http://www.opfocus.com/blog/how-to-watermark-a-visualforce-pdf/ -
Saddam
MemberOctober 14, 2019 at 6:27 am in reply to: do we need to register a lightning application event while handling that event on vf page ?Hi,
A component registers that it may fire an application event by using <aura:registerEvent> in its markup. The name attribute is required but not used for application events. The name attribute is only relevant for component events. This example uses name="appEvent" but the value isn’t used anywhere.
-
Saddam
MemberOctober 14, 2019 at 6:24 am in reply to: What are the different ways to conditionally display markup, the preferred approach in salesforce?Hi,
Using the <aura:if> tag
Use CSS to toggle visibility of markup by calling $A.util.toggleClass(cmp, ‘class’) in JavaScript code -
Saddam
MemberOctober 14, 2019 at 6:00 am in reply to: What is Lightning Design System (SLDS) in salesforce?Hi,
Salesforce released a user interface called Salesforce Lightning Design System as open source. This provides all CSS (Cascading Style Sheets) and Images to create the Standard lightning Component instead of write CSS again for lightning Components. We can build rich enterprise experience and custom applications with the patterns using Salesforce Lightning Design System.
-
Saddam
MemberOctober 11, 2019 at 6:55 am in reply to: How to use External CSS in lightning components?Hi,
you can use this tag
<ltng:require styles=”{! $Resource.staticResourceFileName}”/>
-
Saddam
MemberOctober 11, 2019 at 6:53 am in reply to: Which standard chart type can be placed on the salesforce dashboard?Hi,
You can show data in reports and dashboards in the form of bars, columns, lines, shapes, or other elements. Which is right depends on what the data is about and what you want to show with it.
1.Bar Charts
A bar chart shows values as horizontal lengths, so this format can be good for comparing distance or time. Use a bar chart when you have a summary report with a single grouping, or you only want to display one grouping.
2.Column Charts
A column chart is very much like a bar chart, but it can be a better format for showing relative counts of things, such as leads or dollars. Use a column chart when you have a summary report with a single grouping, or you only want to display one grouping.
3.Line Charts
Line charts are good for showing changes in the value of an item over a series of points in time, such as week to week or quarter to quarter. Use a line chart when you have one important grouping representing an ordered set of data and one value to show.
4.Pie Charts
Use a pie chart when you have multiple groupings and want to show the proportion of a single value for each grouping against the total.
5.Donut Charts
Use a donut chart when you have multiple groupings and want to show not only the proportion of a single value for each grouping against the total, but also the total amount itself.
6.Funnel Charts
Use a funnel chart when you have multiple groupings in an ordered set and want to show the proportions among them.
7.Scatter Charts
Use scatter charts to show meaningful information using one or two groups of report data plus summaries. -
Saddam
MemberOctober 11, 2019 at 5:57 am in reply to: What are the permissions that are set in a user's profile in salesforce?HI,
A permission set is a collection of settings and permissions that give users access to various tools and functions. The settings and permissions in permission sets are also found in profiles, but permission sets extend users’ functional access without changing their profiles.
Users can have only one profile but, depending on the Salesforce edition, they can have multiple permission sets. You can assign permission sets to various types of users, regardless of their profiles.
Create permission sets to grant access among logical groupings of users, regardless of their primary job function. For example, let’s say you have several users with a profile called Sales User. This profile allows assignees to read, create, and edit leads. Some, but not all, of these users also need to delete and transfer leads. Instead of creating another profile, create a permission set.
-
Saddam
MemberOctober 10, 2019 at 6:20 am in reply to: How to show xml document result on visualforce page?Hi,
Inside your controller write a class:
class person{
public String name{get; set;}
public String last_name{get; set;}
//Then you make the constructor for this class
public person(String name, String last_name){
this.name = name;
this.last_name = last_name;
}
}
once you have your class defined (write it on the bottom of the controller code before the last '}' character
create a variable on the top of the controller as you did with public XMLDom.Element person { get; set; }
and then instantiate it. It would something like this:
public XMLDom.Element personXML { get; set; }
public person myPerson{get; set;}
Then once you got the info loaded on personXML just instantiate myPerson by calling its constructor
myPerson = new myPerson(personXML.getValue('Name), personXML.getValue('last_name'));
now all you need is to acces to myPerson on vf page by simply doing this {!myPerson.name} {!myPerson.last_name}
The fields i put are just an example and i changed the name of your variable person to personXML so i could name my class as person
-
Saddam
MemberOctober 10, 2019 at 6:18 am in reply to: How to enable multiple currency in salesforce?Hi,
In Setup, enter Company Information in the Quick Find box, then select Company Information and click Edit. Ensure that your selected currency locale is the default currency that you want to use for current and future records. Enable Activate Multiple Currencies, and then save your changes.
-
Saddam
MemberOctober 10, 2019 at 6:15 am in reply to: Difference between repeat and pageblockTable in salesforce?Hi,
apex:pageBlockTable represents a table formatted and styled to look like a related list table.
apex:repeat allows any arbitrary output based on a template. The four elements require value and var attributes, iterate over a collection of some sort, may generally be nested inside each other, and are limited to 1,000/10,000 rows of output, depending on the apex:page's readOnly attribute.
-
Hi,
When you use 'with sharing'(with Security Settings enforced) keyword the Apex code runs in User Context, i.e all the sharing rules for the current user are taken into account.
When you use 'without sharing' keyword the Apex code runs in system context, Apex code has access to all objects and fields— object permissions, field-level security, and sharing rules aren’t applied for the current user.