Popular Salesforce Blogs
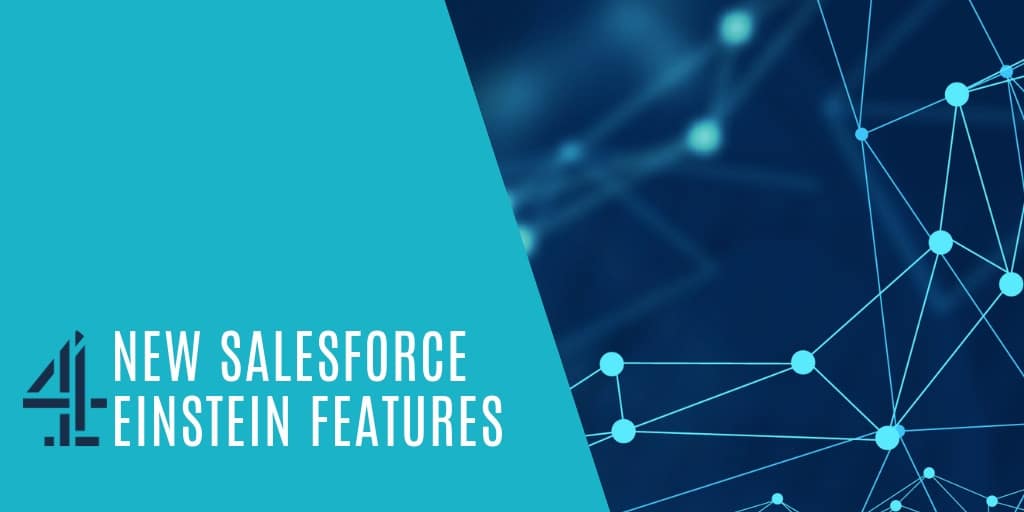
4 New Salesforce Einstein Features – What it means for Service Cloud users
Salesforce Einstein Key Features – Salesforce’s recent announcement of Einstein Artificial Intelligence capabilities have quickly made headlines and for all the right reasons. With the…

5 Groundbreaking Features Exclusive to Salesforce Lightning Experience
With Salesforce stopping to make enhancements to Salesforce Classic, companies have started moving to Salesforce Lightning Experience. However, migrating from Salesforce Classic to Salesforce Lightning…

Easy Way to Integrate Salesforce CLI with Visual Studio Code
What is Salesforce CLI? Salesforce CLI is a command-line interface that simplifies development and builds automation when working together with your Salesforce org. Use it…
Popular Salesforce Videos
How Salesforce Industries can Help Organizations to Save Millions?
Salesforce Industries i.e Vlocity empowers businesses with powerful platforms to boost collaboration and makes it easy to deliver great customer experiences. It provides tailored solutions…
What are Collections in Apex? | Salesforce Development Course
Shrey explained different types of Collections in Apex in this video. Watch the video now and learn, let us know in the comments if you…
Sharing Rules in Salesforce
Use sharing rules to extend sharing access to users in public groups, roles, or territories. Sharing rules allow administrators to automatically bypass organization-wide sharing settings.…