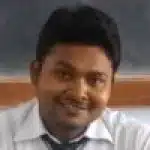
Shaharyar Ansari
IndividualForum Replies Created
-
Shaharyar
MemberAugust 9, 2017 at 12:09 pm in reply to: how to integrate salesforce with MongoDB without any tools??'Most of MongoDB to Salesforce integration practice is about making custom REST API solutions to access mongoDB JSON files, and pull,push, or delete data from Salesforce databases.
Here are some steps to integrate it(MongoDB) with SalesForce.
1.Authentication
This step involves authenticating yourself for accessing MongoDB databases, and also Salesforce databases, if you are planning a migration that is. Yes, pretty basic stuff.
- 2.Using MongoDB API for data access:
- MongoDB uses its own ‘Database format’, BSON, which is a modified form of JSON. Though database format is not strictly an accurate term for BSON but for simplicity we can use the same as long as it serves the purpose.MongoDB has its own set of APIs to access its BSON data. For accessing the databases you would basically need a JavaScript program that can use MongoDB BSON HTTP interfaces to pull and if required, push and delete data collections, or documents, or data etc. As said it is a custom program, hence no plug-and-play solution.
- 3.REST API solution for accessing the salesforce.
- Now once you have retrieved the MongoDB data, you will have to create you own REST API solution to push that data into Salesforce interface or Salesforce database.
-
Shaharyar
MemberAugust 8, 2017 at 5:07 am in reply to: When we will use of GET and POST in the Integration Services?# POST is used to create record using web services:
# Whereas GET is used to retrieve record.
# Example can be seen as:
Method :- Get
URL:- /services/data/v36.0/sobjects/Account/0019000001hE8afMethod :- Post
URL:- /services/data/v36.0/sobjects/Account/
Request Body :-{
"Name" : "Shaharyar4U",
"phone" : "7867867860",
"website" : "www.mysite.com",
"numberOfEmployees" : "786",
"industry" : "Computer"
} -
<apex:param> tag is mainly used to pass values from JavaScript to an Apex controller, it can only be used with the following parent tags/The <apex:param> component can only be a child of the following components:.
• <apex:actionFunction>
• <apex:actionSupport>
• <apex:commandLink>
• <apex:outputLink>
• <apex:outputText>
• <flow:interview>- One example can be seen as:
#VisualForce Page:
<apex:page controller="paramtest" docType="html-5.0">
<apex:form><apex:commandbutton action="{!testdirect}" reRender="test" value="Static value">
<apex:param assignTo="{!value}" value="The static value that was set from vf page"/>
</apex:commandbutton><br/>
<input type="text" id="testinput"/>
<input type="button" onclick="testinputJS()" value="Dynamic Value" class="btn"/>
<apex:outputPanel id="test">
<apex:outputText value="{!value}"/>
</apex:outputPanel>
<apex:actionFunction action="{!testinput}" name="passToController" rerender="test">
<apex:param value="" name="inpval"/>
</apex:actionFunction>
</apex:form>
<script>
function testinputJS(){
var str = document.getElementById('testinput').value;
if(str.length >4){
str= str.substring(0,4);
}
passToController(str);
}
</script>
</apex:page>#Apex Class/Controller:
public class paramtest {
public string value { get; set;}public void testdirect(){
system.debug(value);
}
public void testinput(){
value = apexpages.currentPage().getParameters().get('inpval');
system.debug(value);
}
} -
Shaharyar
MemberAugust 4, 2017 at 9:49 am in reply to: How to add Custom Field on Particular Object through Salesforce Apex Class?Please try this it will work.
public static void createObject()
{
MetadataService.MetadataPort service = createService();
MetadataService.CustomObject customObject = new MetadataService.CustomObject();
customObject.fullName = 'Test__c';
customObject.label = 'Test';
customObject.pluralLabel = 'Tests';
customObject.nameField = new MetadataService.CustomField();
customObject.nameField.type_x = 'Text';
customObject.nameField.label = 'Test Record';
customObject.deploymentStatus = 'Deployed';
customObject.sharingModel = 'ReadWrite';
List<MetadataService.SaveResult> results =
service.createMetadata(
new MetadataService.Metadata[] { customObject });
handleSaveResults(results[0]);
}public static void createField()
{
MetadataService.MetadataPort service = createService();
MetadataService.CustomField customField = new MetadataService.CustomField();
customField.fullName = 'Test__c.TestField__c';
customField.label = 'Test Field';
customField.type_x = 'Text';
customField.length = 42;
List<MetadataService.SaveResult> results =
service.createMetadata(
new MetadataService.Metadata[] { customField });
handleSaveResults(results[0]);
} -
Shaharyar
MemberAugust 4, 2017 at 9:23 am in reply to: What is RestResource Annotation in Salesforce?- REST annotation enables you to expose an Apex class or an Apex method as a REST resource. In salesforce, there are six REST annotations, that is :
1). @RestResource(urlMapping=’/your url’)
2). @HttpGet
3). @HttpPost
4). @HttpPut
5). @HttpPatch
6). @HttpDelete
7). @HttpHead
- Classes with REST annotation should be defined as global.
- Methods with REST annotation should be defined as global static.
I am giving you 1 example of HttpGet...
@HttpGet is used at method level. This method is called when a HTTP GET request is sent. To expose your apex class as REST resource you should use @RestResource(urlMapping=’/your url’) annotation at class level as shown below.
@RestResource(urlMapping='/your_url')
global class restExample {@HttpGet
global static void exampleMethod() {// Write your code here
}
}Here url mapping is relative to https://instance.salesforce.com/services/apexrest/.
- If your class is in namespace say ‘abc’, then API url must be as follows:
- https://instance.salesforce.com/services/apexrest/abc/you_url
URL are case-sensitive.
-
Shaharyar
MemberJune 16, 2017 at 7:19 am in reply to: How can i display records of account, contacts, opty on the same Salesforce Visualforce page and then process the selected records using wrapper class?/*VISUAL FORCE PAGE AND CONTROLLER WITH WRAPPER CLASS*/
<apex:page controller="MultipleContact" sidebar="false">
<apex:form >
<apex:pageBlock >
<apex:pageBlockTable value="{!listContact}" var="cnct" id="ref">
<apex:column headervalue="Last Name">
<apex:inputfield value="{!cnct.con.LastName}"/>
</apex:column>
<apex:column headervalue="First Number">
<apex:inputfield value="{!cnct.con.FirstName}"/>
</apex:column>
<apex:column headervalue="Email">
<apex:inputfield value="{!cnct.con.Email}"/>
</apex:column>
<apex:column headervalue="Title">
<apex:inputfield value="{!cnct.con.title}"/>
</apex:column>
<apex:column headervalue="RemoveContact">
<apex:inputCheckbox value="{!cnct.isSelected}"/>
</apex:column>
</apex:pageBlockTable>
<apex:pageblockbuttons >
<apex:commandButton value="Add More Contact" action="{!addContact}" reRender="ref" />
<apex:commandButton value="Save Contact" action="{!SaveContact}"/>
<apex:commandButton value="Remove Contact" action="{!RemoveContact}"/>
</apex:pageblockbuttons>
</apex:pageBlock>
</apex:form>
</apex:page>
public class MultipleContact{public list<cContact> listContact{get;set;}
public MultipleContact(){ //constructor
listContact=new list<cContact>();
listContact.add(new cContact(new contact()));
}
public void addContact() {
Contact cnct = new Contact();
MultipleContact.cContact cn = new MultipleContact.cContact(cnct);
listContact.add(cn);
}
public pagereference saveContact(){
List<Contact> myList = new List<Contact>();
for(cContact c : listContact){
myList.add(c.con);
}
pagereference pg=new pagereference('/003/o');
insert myList;
return pg;
}
public pageReference RemoveContact() {
List<cContact> li = listContact;
for(Integer i=0 ; i<li.size(); i++){
if(li[i].isSelected == true){
listContact.remove(i);
}
}
pageReference pg1=new pageReference('/');
//delete listContact;
return null;
}
//Inner class of contact
public class cContact{
public Contact con{get;set;}
public boolean isSelected{get;set;}
public cContact(Contact con){
this.con = con;
isSelected = false;
}
}}