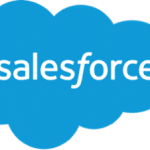
shariq
IndividualForum Replies Created
-
shariq
MemberAugust 14, 2017 at 1:55 pm in reply to: How To add link on the Account Name for below given Scenario ?Hi Aman,
You can try this:-
Apex Controller
public class AccountLink
{
public String linkId {get;set;}
public List<Contact> con{get;set;}
public String searchString{get;set;}
public List<Account> acct{get;set;}public void search1()
{acct= Database.query('Select Name, description From Account WHERE Name LIKE \'%'+searchString+'%\' Limit 100');
con = new List<contact>();
}public void link()
{con = [SELECT Id, Description, AccountId FROM Contact WHERE AccountId =:linkId LIMIT 100];
}
}Apex Page
<apex:page controller="AccountLink" sidebar="false" id="page">
<apex:form >
<apex:actionFunction action="{!search1}" name="callingApex" reRender="idBlock1"/>
<apex:pageBlock Id="idBlock">
<apex:inputText value="{!searchString}" onkeyup="callApex()" />
<apex:pageBlockButtons >
<apex:commandButton value="search" action="{!search1}" rerender="idBlock1"/>
</apex:pageBlockButtons><apex:pageBlockTable value="{!acct}" var="account" id="idBlock1">
<apex:column headerValue="Account Name" >
<apex:commandLink value="{!account.name}" action="{!Link}" reRender="idPage">
<apex:param value="{!account.Id}" name="ContactsShow" assignTo="{!linkId}" />
</apex:commandLink>
</apex:column>
<apex:column ><apex:pageBlockTable value="{!con}" var="contact" id="idPage">
<apex:column value="{!contact.Description}"/>
<apex:column value="{!contact.Id}"/>
</apex:pageBlockTable></apex:column>
</apex:pageBlockTable>
</apex:pageBlock>
</apex:form>
<script>
function callApex()
{
callingApex();
}
</script>
</apex:page>Hope this helps.
-
Hi Saloni,
You can try this:-
<apex:pageBlockTable var="customAccount" value="{!mapIntVsAcc}" id="pageId">
<apex:column ><h1>
Account Name
</h1> <apex:inputfield value="{!mapIntVsAcc[customAccount].Name}" /></apex:column></apex:pageBlockTable>
customAccount will give you the key of map.
Hope this helps.
-
Hi Pawan,
The trigger provided below will work for newly created account and contact's records, set the default value 0 for the two number fields created on account. This trigger will work for insert/update/delete on contact.
trigger Count_MalesAndFemales on Contact (after insert, after update, before delete)
{
//System.debug('ggg'+Trigger.Old[0].AccountId);
List<Account> accList = new List<Account>();
Map<Id,Contact> mapIdVsCon = new Map<Id,Contact>();if(Trigger.isAfter&&Trigger.isInsert)
{
List <Contact> conListNew = [SELECT Gender__c, AccountId, Account.news__No_Of_Males__c, Account.news__No_Of_Females__c FROM Contact WHERE AccountId !=Null And Id IN : Trigger.new ];
for(Contact con : conListNew)
{
if(con.Gender__c == 'Male')
{
con.Account.No_Of_Males__c++;
System.debug('sss'+con.Account.No_Of_Males__c);
}
else if(con.Gender__c == 'Female')
{
con.Account.No_Of_Females__c++;
System.debug('hhh'+con.Account.No_Of_Females__c);
}
mapIdVsCon.put(con.AccountId,con);
//accList.add(acc);
}
}if(Trigger.isAfter&&Trigger.isUpdate)
{
List <Contact> conListNew = [SELECT Gender__c, AccountId, Account.news__No_Of_Males__c, Account.news__No_Of_Females__c FROM Contact WHERE AccountId !=Null And Id IN : Trigger.new ];
for(Contact con : conListNew)
{
if(Trigger.newMap.get(con.Id).Gender__c == 'Male' && Trigger.oldMap.get(con.Id).Gender__c == 'Female')
{
con.Account.No_Of_Females__c--;
con.Account.No_Of_Males__c++;
}
else if(Trigger.oldMap.get(con.Id).Gender__c == 'Male' && Trigger.newMap.get(con.Id).Gender__c == 'Female')
{
con.Account.No_Of_Females__c++;
con.Account.No_Of_Males__c--;
}
mapIdVsCon.put(con.AccountId,con);
//accList.add(acc);
}
}if(Trigger.isBefore&&Trigger.isDelete)
{
List <Contact> conListOld = [SELECT Gender__c, AccountId, Account.news__No_Of_Males__c, Account.news__No_Of_Females__c FROM Contact WHERE AccountId !=Null And Id IN : Trigger.old ];
for(Contact con : conListOld)
{
System.debug('sss'+con);
System.debug('sss'+Trigger.oldMap.get(con.Id));
if(con.Gender__c == 'Female')
{
System.debug('sss'+Trigger.oldMap.get(con.Id));
con.Account.No_Of_Females__c--;
}
else if(con.Gender__c == 'Male' )
{
System.debug('sss'+Trigger.oldMap.get(con.Id));
con.Account.No_Of_Males__c--;
}
mapIdVsCon.put(con.AccountId,con);
//accList.add(acc);
}
}for(Id ide : mapIdVsCon.keySet())
{
Account a = new Account();
a.Id = ide;
a.news__No_Of_Males__c = mapIdVsCon.get(ide).Account.news__No_Of_Males__c;
a.news__No_Of_Females__c = mapIdVsCon.get(ide).Account.news__No_Of_Females__c;
accList.add(a);
System.debug('key===='+ide);
System.debug('id====='+mapIdVsCon.get(ide));
}
update accList;
} -
shariq
MemberAugust 11, 2017 at 1:24 pm in reply to: Can we call apex function and script function at the same time by clicking a button on Salesforce visualforce?Thanks Radhakrishna,
This helps a lot.
-
shariq
MemberAugust 10, 2017 at 2:08 pm in reply to: Why we use Test.starTest and Test.stopTest in Salesforce?Hi Shaharyar,
Test.startTest and Test.stopTest are used for asynchronous apex, like Batch Apex and Future calls. Calling method(s) between Test.startTest and Test.stopTest ensure that any asynchronous transactions finish executing before Test.stopTest() exits. This means, that if you use System.asserts after Test.stopTest, you'll have an accurate representation of your test. Otherwise, if you called System.assert before Test.stopTest, or if you didn't use Test.startTest / Test.stopTest, then you couldn't gaurantee that the batch or future transaction was complete.
startTest()
Marks the point in your test code when your test actually begins. Use this method when you are testing governor limits.
Usage
You can also use this method with stopTest to ensure that all asynchronous calls that come after the startTest method are run before doing any assertions or testing. Each test method is allowed to call this method only once. All of the code before this method should be used to initialize variables, populate data structures, and so on, allowing you to set up everything you need to run your test. Any code that executes after the call to startTest and before stopTest is assigned a new set of governor limits.
stopTest()
Marks the point in your test code when your test ends. Use this method in conjunction with the startTest method.
Usage
Each test method is allowed to call this method only once. Any code that executes after the stopTest method is assigned the original limits that were in effect before startTest was called. All asynchronous calls made after the startTest method are collected by the system. When stopTest is executed, all asynchronous processes are run synchronously.
Hope this helps.
-
Hi Aman,
A wrapper or container class is a class, data structure, or an abstract data type whose instances are a collections of other objects.It is a custom object defined by Salesforce developer where he defines the properties of the wrapper class. Within Apex & Visualforce this can be extremely helpful to achieve many business scenarios within the Salesforce CRM software.
Using Wrapper classes we can have the ability to check few records from the displayed list and process them for some action.
Here is the Example.
Hope this helps.
-
shariq
MemberAugust 9, 2017 at 12:18 pm in reply to: Updating Rollup Summary field executes the parent object trigger?Hi Himanshu,
Update Trigger is fired when there is any change in the field of that sobject, so whenever child updates, the roll up summary field on parent sobject updates which will fire the trigger.
Hope this helps.
-
shariq
MemberAugust 8, 2017 at 1:57 pm in reply to: What is the purpose or use of @TestVisible in salesforce? -
shariq
MemberAugust 8, 2017 at 1:50 pm in reply to: How to update lookup field from another lookup field in Salesforce?Hi Bhanu Prakash Reddy,
You can write a trigger on the object which is containing first LookUp field.
-
Thanks Shaharyar,
It helps a lot.
-
shariq
MemberAugust 7, 2017 at 12:52 pm in reply to: How can we write a trigger for before and after DML operation?Hi Mohit,
Before triggers can be used to update or validate record values before they are saved to the database.
After triggers can be used to access field values that are set by the database (such as a record's Id or lastUpdated field) and to affect changes in other records, such as logging into an audit table or firing asynchronous events with a queue.
Use Before Trigger:
In the case of validation check in the same object.
Insert or update the same object.Use After Trigger:
Insert/Update related object, not the same object.
Notification email.
We cannot use After trigger if we want to update a record because it causes read only error. This is because, after inserting or updating, we cannot update a record.trigger AccountTrigger on Account (before insert, after insert)
{
if(Trigger.isBefore)
{
if(Trigger.IsInsert)
{
Contact cont = new Contact();
cont.LastName = Trigger.new[0].name;
cont.FirstName = Trigger.new[0].name;
insert cont;
}
else if(Trigger.IsInsert&&Trigger.IsAfter)
{
Contact cont = new Contact();
cont.LastName = '(Updated)';
cont.FirstName = Trigger.new[0].name+ 'Updated';
insert cont;
}
}
} -
shariq
MemberAugust 4, 2017 at 10:16 am in reply to: How can we Delete mass Accounts from org using Dml operation in salesforce?Hi Vikas,
You may use Batch Class to delete all records of Account sobject.
For Example :-
Global class BatchMassDeleteRecs Implements Database.batchable<sobject>{
global final string query;
global BatchMassDeleteRecs(string q){query=q;
}global Database.QueryLocator start(Database.BatchableContext BC){
return Database.getQueryLocator(query);
}
global void execute(Database.BatchableContext BC,List<SObject> scope){
delete scope;}
global void finish(Database.BatchableContext BC){
}}
-
Hi Suraj,
You can't delete a user, but you can deactivate an account so a user can no longer log in to Salesforce. From Setup, enter Users in the Quick Find box, then select Users. Deselect the Active checkbox and then click Save.
-
shariq
MemberAugust 4, 2017 at 9:42 am in reply to: What is WhoId and WhatId in Salesforce activities?Hi Manpreet,
WhatId :-
It refers to objects.
ID of a related Account, Opportunity, Campaign, Case, or custom object. Label is Opportunity/Account ID.
WhoId :-
It refers to people.
ID of a related Contact or Lead. If the WhoId refers to a lead, then the WhatId field must be empty. Label is Contact/Lead ID. If Shared Activities is enabled, this is the ID of a related Lead or primary Contact.
-
Thanks Shaharyar,
It helps a lot.
-
shariq
MemberAugust 3, 2017 at 12:14 pm in reply to: What is the difference between Record-Level Security and Field-Level Security in Salesforce?Hi Aman,
Record Level Security :-
Record-level security lets you give users access to some object records, but not others. Every record is owned by a user or a queue. The owner has full access to the record. In a hierarchy, users higher in the hierarchy always have the same access to users below them in the hierarchy. This access applies to records owned by users, as well as records shared with them.
Field Level Security :-
Field-level security settings let you restrict users’ access to view and edit specific fields. Your Salesforce org contains a lot of data, but you probably don’t want every field accessible to everyone. For example, your payroll manager probably wants to keep salary fields accessible only to select employees.
Hope this helps.
-
Hi sfdc,
Check your Child Relationship Name, you can check by following steps :-
1. Go to the view fields section of that sobject.
2.Click the field label that you want to check(Master Detail or LookUp field).
3.There you will get the Child Relationship Name, use it to your query.
Hope this helps.
-
shariq
MemberAugust 2, 2017 at 1:42 pm in reply to: What are the different types of sharing in salesforce ?Hi Aman,
Force.com Managed Sharing :-
Force.com managed sharing involves sharing access granted by Force.com based on record ownership, the role hierarchy, and sharing rules:-
Record Ownership:-
Each record is owned by a user or optionally a queue for custom objects, cases and leads. The record owner is automatically granted Full Access, allowing them to view, edit, transfer, share, and delete the record.
Role Hierarchy:-
The role hierarchy enables users above another user in the hierarchy to have the same level of access to records owned by or shared with users below. Consequently, users above a record owner in the role hierarchy are also implicitly granted Full Access to the record, though this behavior can be disabled for specific custom objects. The role hierarchy is not maintained with sharing records. Instead, role hierarchy access is derived at runtime. For more information, see “Controlling Access Using Hierarchies” in the Salesforce online help.For more information you can refer to this link.
-
shariq
MemberAugust 2, 2017 at 12:41 pm in reply to: Difference between global and static keyword in Salesforce?Hi Shubham,
Static Keyword :-
Static variables are variables that belong to an overall class, not a particular object of a class. Think of a static variable to be like a global variable – its value is shared across your entire org. Any particular object’s properties or values are completely irrelevant when using static. Similarly, are methods that act globally and not in the context of a particular object of a class.
Global Keyword :-
This means the method or variable can be used by any Apex code that has access to the class, not just the Apex code in the same application. This access modifier should be used for any method that needs to be referenced outside of the application, either in the SOAP API or by other Apex code. If you declare a method or variable as global, you must also declare the class that contains it as global.
-
shariq
MemberAugust 1, 2017 at 12:34 pm in reply to: Where do we use apex:detail and apex:inlineeditsupport in Salesforce?Hi Shubham,
apex:inlineeditsupport :-
This component provides inline editing support to <apex:outputField> and various container components. In order to support inline editing, this component must also be within an <apex:form> tag.
The <apex:inlineEditSupport> component can only be a descendant of the following tags:
<apex:dataList>
<apex:dataTable>
<apex:form>
<apex:outputField>
<apex:pageBlock>
<apex:pageBlockSection>
<apex:pageBlockTable>
<apex:repeat>apex:detail :-
The standard detail page for a particular object, as defined by the associated page layout for the object in Setup. This component includes attributes for including or excluding the associated related lists, related list hover links, and title bar that appear in the standard Salesforce application interface.
For more information you can refer to link1, link2.
Hope this helps.
-
shariq
MemberAugust 1, 2017 at 10:00 am in reply to: What is the difference between ISBLANK() AND ISNULL() in salesforce? -
shariq
MemberAugust 1, 2017 at 7:14 am in reply to: What is the difference between the “Salesforce” and “Salesforce Platform” licenses?Hi Suraj,
If a user only needs to access custom Force.com or AppExchange apps, it's OK to assign them a Salesforce Platform license. On the other hand, if a user needs access to any CRM functionality (eg Sales or Service Cloud), they must be assigned a Salesforce license.
For more Information You can refer to this link.
Hope this helps.
-
shariq
MemberJuly 31, 2017 at 6:29 am in reply to: What is the difference between insert() and database.insert() in Salesforce? -
shariq
MemberJuly 31, 2017 at 6:20 am in reply to: How can I change the width / height of an apex:inputField on a VisualForce page?Hi Tanu,
You can try this :-
<apex:inputField value="Input Test" style="width: 360px;height: 40px"/>
Hope this helps.
-
shariq
MemberJuly 31, 2017 at 6:16 am in reply to: Can we create a collapsible pageblock table or Html table on a visualforce page?Hi Ajit,
You can create collapsible pageblock table using pageblock section:-
<!-- Page: -->
<apex:page standardController="Account">
<apex:form>
<apex:pageBlock title="My Content" mode="edit">
<apex:pageBlockButtons>
<apex:commandButton action="{!save}" value="Save"/>
</apex:pageBlockButtons>
<apex:pageBlockSection title="My Content Section" columns="2" collapsible="true">
<apex:inputField value="{!account.name}"/>
<apex:inputField value="{!account.site}"/>
<apex:inputField value="{!account.type}"/>
<apex:inputField value="{!account.accountNumber}"/>
</apex:pageBlockSection>
</apex:pageBlock>
</apex:form>
</apex:page>
Hope this helps