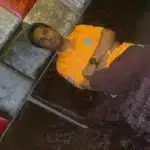
Sourabh Goyal
IndividualForum Replies Created
-
Sourabh
MemberNovember 7, 2016 at 10:28 am in reply to: Can I Insert a Static Resource using Apex Code in Salesforce?Hi Ajit,
Metadata Api can help you out for creating static resource, Do someting like this:-
MetadataService.MetadataPort service = createService();
MetadataService.StaticResource staticResource = new MetadataService.StaticResource();
staticResource.fullName = 'MyResource';
staticResource.contentType = 'text';
staticResource.cacheControl = 'public';
staticResource.content = EncodingUtil.base64Encode(Blob.valueOf('Static stuff'));
MetadataService.AsyncResult[] results = service.create(new List<MetadataService.Metadata> { staticResource });Thanks
-
Sourabh
MemberSeptember 15, 2016 at 12:30 pm in reply to: How to get Custom field id in Salesforce?Hi Tanu,
To get custom field Id in Salesforce you have to use Tooling Api. Here is a piece of code , Hopefully it will help you.
HttpRequest req = new HttpRequest();
req.setHeader('Authorization', 'Bearer ' + UserInfo.getSessionID());
req.setHeader('Content-Type', 'application/json');String toolingendpoint = 'https://na1.salesforce.com/services/data/v20.0/tooling/';
//query for custom fields
endpoint += 'query/?q=select+id,DeveloperName,FullName+from+CustomField+limit+1'
req.setEndpoint(endpoint);
req.setMethod('GET')Http h = new Http();
HttpResponse res = h.send(req);
system.debug(res.getBody());Thanks
-
Sourabh
MemberJuly 27, 2016 at 2:19 pm in reply to: How can I change the width / height of an apex:inputField on a VisualForce page?Hi Tanu,
<apex:inputfield> tag contains style element in which you can put styling as you needed.
example: <apex:inputfield value="{!value from controller}" style="height:100px;width:100px">
Thanks..
-
Sourabh
MemberJuly 27, 2016 at 2:06 pm in reply to: Is there any way to can create the profile in the salesforce in apex test class. How?Hi Mohit,
You cannot create profiles in apex code, either through tests or otherwise, this is presumably a security limitation.
Thanks
-
Sourabh
MemberJuly 25, 2016 at 12:17 pm in reply to: How to show PickList field as Radio Buttons on Visualforce Page?Hi Tanu,
Try this code , Hope so it will be helpful for you..
public List<SelectOption> getTypes(){
Schema.sObjectType sobject_type = customObject__c.getSObjectType();Schema.DescribeSObjectResult sobject_describe = sobject_type.getDescribe();
Map<String, Schema.SObjectField> field_map = sobject_describe.fields.getMap();
List<Schema.PicklistEntry> pick_list_values = field_map.get('picklistField__C').getDescribe().getPickListValues();
List<selectOption> options = new List<selectOption>();
for (Schema.PicklistEntry a : pick_list_values) {
options.add(new selectOption(a.getLabel(), a.getValue()));
}
return options;to use this on page:
<apex:selectRadio value="{!customObject__c.picklistField__c}">
<apex:selectoptions value="{!types}"></apex:selectoptions>
</apex:selectRadio>
Here picklistField__c is a field name whhich contains picklist values..Thanks
-
Sourabh
MemberJuly 25, 2016 at 6:59 am in reply to: How can we get the distinct account name of account object on Salesforce?Hi Mohit,
Giving you the code for getting distinct Account name using 'set'...
List<Account> acclist = new List<Account>();
Set<String> setName = new Set<String>();
acclist = [select id,Name other fields from Account] ;
for (Integer i = 0; i< acclist.size(); i++)
{
setName.add(acclist[i].Name); // It will contains distinct accounts.
}
Thanks
-
Sourabh
MemberJuly 25, 2016 at 5:55 am in reply to: Is there any way to perform arithematic operation using Apex tags on page itself?Hi Mohit,
For performing arithematic operations on page itself you can use javascript/jquery or there is an apex tag named 'Apex:variable' which can be very handy for performing simple arithematic operations on visual force pages.
Thanks
-
Sourabh
MemberJuly 25, 2016 at 5:52 am in reply to: Is there any way to perform DML operation on external database and how?Hi Mohit,
For performing DML operations on external systems, first you have to integrate with that system. You can use either REST api or SOAP api to integrate with external system.
After successfully integration you can perform the DML operations.
Thanks
-
Sourabh
MemberJuly 20, 2016 at 11:18 am in reply to: How can I show report or dashboard on vf page?Hi Pranav,
If you want to add standard reports/dashboards to a VF page, you have to use iframe for it.
You can set the source of the iframe to the URL of your report/dashboard.eg.
<apex:page >
<apex:iframe src="url of your report/dashboard" scrolling="true" id="theIframe"/>
</apex:page>Thanks
-
Sourabh
MemberJuly 19, 2016 at 1:11 pm in reply to: How can we write a such apex controller class which can be used by multiple vf pages on requirement?Hi Shekar,
Actually i have written it incorrect, sorry for that.
If you are not using StandardController do:-
<apex:page controller=”ControllerName”>
Thanks.
-
Sourabh
MemberJuly 19, 2016 at 1:06 pm in reply to: How to create a vf page automatically on creation of Account?Hi Shekhar,
I am providing you an example of Dynamic Page creation using ApexTooling Api.*Here i have created a vf page in which the object name is entered(custom/standard) on entering object name in input field & clicking on 'create page', a page is been created dynamically refrencing to the object in StandardController.
* Make sure you have put your base url in Remote Site Settings, It is needed for callout.
Vf Page:-
<apex:page controller="ctrl_DynamicPageCreation">
<apex:form id="form1"><div style="margin-left: 23px;">
<h1 style="padding-right: 10px;"> Object Name</h1>
<apex:inputText html-placeholder="Object Name..." value="{!objectName}">
</apex:inputText>
</div> <br/>
<apex:commandButton value="CreatePage" action="{!createpage}" reRender="Error" style="margin-left: 23px;"/>
</apex:form><apex:outputpanel id="Error">
<apex:pageMessages ></apex:pageMessages>
</apex:outputpanel>
</apex:page>
Controller:-public class ctrl_DynamicPageCreation{
public String objectName{get;set;}
Public String name;public ctrl_DynamicPageCreation(){}
public void createpage(){
if(objectName.contains('__c')){
name = objectName.replace('__c','');
}system.debug('****'+objectName);
String salesforceHost = System.Url.getSalesforceBaseURL().toExternalForm();String url = salesforceHost + '/services/data/v29.0/sobjects/ApexPage';
HttpRequest req = new HttpRequest();
req.setMethod('POST');
req.setEndpoint(url);
req.setHeader('Content-type', 'application/json');
req.setHeader('Authorization', 'Bearer ' + UserInfo.getSessionId());
//for controllerType = >0 -- no controller
//req.setBody('{"Name" : "TestPageFromRest","Markup" : "<apex:page>hello</apex:page>","ControllerType" : "0","MasterLabel":"TestPageFromRest","ApiVersion":"29.0"}');//for controllerType => 1 -- Standard controller + extensions
req.setBody('{"Name" : "FileUploader'+name+'","Markup" : "<apex:page standardController=\''+objectName+'\' extensions=\'dynamicCreationController\'>hello</apex:page>","ControllerType" : "1","MasterLabel":"FileUploader'+objectName+'","ApiVersion":"29.0"}');//for controllerType => 3 --custom Controller
//req.setBody('{"Name" : "DynamicPage'+objectName+'","Markup" : "<apex:page controller=\''+objectName+'\'>hello</apex:page>","ControllerType" : "3","MasterLabel":"TestPageFromRestCase23","ApiVersion":"29.0"}');
Http http = new Http();HTTPResponse res = http.send(req);
System.debug(res.getBody());
if(string.valueof(res.getBody()).contains('success')){
ApexPages.Message myMsg = new ApexPages.Message(ApexPages.Severity.CONFIRM,'Your Page has been created..');
ApexPages.addMessage(myMsg);
}
else{
string errorMessage = string.valueof(res.getBody());
string test = errorMessage.substring(13,errorMessage.indexOf('","errorCode'));
system.debug('&&&&'+test);
if(test.contains('Markup')){
ApexPages.Message myMsg = new ApexPages.Message(ApexPages.Severity.ERROR,'Object does not exist. Please check the spelling and try again.');
ApexPages.addMessage(myMsg);
}else if(test.contains('That page name is already in use, please choose a different one.')){
ApexPages.Message myMsg = new ApexPages.Message(ApexPages.Severity.ERROR,'Page for this object is already been created. Try to choose different one.');
ApexPages.addMessage(myMsg);
}
else{
ApexPages.Message myMsg = new ApexPages.Message(ApexPages.Severity.ERROR,test);
ApexPages.addMessage(myMsg);
}
}
}
}Hope so this information is helpful to you.
Thanks -
Sourabh
MemberJuly 19, 2016 at 9:15 am in reply to: How can I get multi functionality of two buttons in single button using controller extensions?Hi Pranav,
You can use 'action' and 'onclick' respectively on a single button click.
For eg.
<apex:commandButton action="{!savePDF}" onclick="CaptureSignature();" value="Save" id="theButton" />
This command button doing two different functionality on a single click ie. saving pdf as well as capturing signature.Thankyou
-
Sourabh
MemberJuly 19, 2016 at 9:07 am in reply to: How can we write a such apex controller class which can be used by multiple vf pages on requirement?Hi Tanu,
A single apex controller can be used for multiple VisualForce pages as per need. You just have to give the reference of the controller in your page.
If you are using StandardController do like this:-
<apex:page Standardcontroller="Account" extensions="ControllerName">
If you are not using StandardController do:-
<apex:page Standardcontroller="ControllerName">
Thankyou
-
Sourabh
MemberJuly 19, 2016 at 9:00 am in reply to: How to create a vf page automatically on creation of Account?Hi Tanu,
*For this you have to create a class using ApexTooling Api which create page dynamically. It can be done using REST Api callout used in Tooling Api.
*After this you have to create a trigger which calls the class after insertion of Account.Thankyou
-
Sourabh
MemberJuly 18, 2016 at 9:00 am in reply to: Is there any limitation on adding number of screens in flows?Hello Tanu,
For the governer limits for flows in salesforce you can visit:- https://help.salesforce.com/apex/HTViewHelpDoc?id=vpm_admin_flow_limits.htm
-
Sourabh
MemberJuly 15, 2016 at 2:27 pm in reply to: Can I set permission set on the Custom Object in Salesforce?Yes you can set permission set on the Custom Object in Salesforce.
1. Go to permission sets.
2. Click on permission set for which object settings should be saved.
3. Go to Object settings, here you will find the objects created in your org.
4. Click on the object(standard/custom) you want to change and edit it.Thankyou
-
Sourabh
MemberJuly 15, 2016 at 1:47 pm in reply to: Disable commandButton after first click to prevent double submissionYou can use javascript for disabling button after the click. Here is a piece of code for that.
<apex:commandButton value="Submit" id="Submit" onClick="SubmitOnClick(this);" />
<apex:actionFunction name="doSubmit" action="{!Submit}" />
</apex:form><script language="Javascript">
function SubmitOnClick (objSubmitBtn) {
objSubmitBtn.disabled = true;
objSubmitBtn.value = 'Submitting...';
doSubmit();
}
</script> -
Sourabh
MemberJuly 15, 2016 at 12:30 pm in reply to: Where a link may open on lightning app as its a one page app?You would do this using the e.force:navigateToComponent method. Try this
In your markup
<ui:button label="ATTENDANCE" press="{!c.navigateToRollCall}"/>
In your controller, do something like
navigateToRollCall : function(component, event, helper) {
var evt = $A.get("e.force:navigateToComponent");
evt.setParams({
componentDef: "c:AttendanceRollCall",
componentAttributes: {
programId: component.get("v.program.Id")
}
});
evt.fire();
}
Thankyou
-
Sourabh
MemberJuly 15, 2016 at 10:52 am in reply to: Permission set is assigned to user, how does it assign to a particular profile not user in Salesforce?Hi Pranav,
The permission sets can only be assigned at a user level.
For more information you may check this link:- https://success.salesforce.com/answers?id=90630000000ggufAAA
Thankyou
-
Sourabh
MemberJuly 15, 2016 at 10:28 am in reply to: How we can we migrate the profile setting of one org to the another profile in another org?Hi Mohit,
You cannot send profile settings directly to another org. For this you have to choose the component and the profile simentaneously if you want that the particular profile for the component will be added automatically while sending it to another org.
For change set:-
1. Add 'Change Set Components'.
2. From 'Profile Settings For Included Components' add profile for which you need the changes to be made.For Ant Script:-
* In package.xml do like this..<Package>
<types>
<members>Component</members>
<name>ComponentName</name><members>Profile</members>
<name>ProfileName</name></types>
<version>36.0</version>
</Package>Thankyou
-
Sourabh
MemberApril 14, 2016 at 8:10 am in reply to: How can I use Custom Pagination in Salesforce?Hi Shubham ,
Giving you an example of custom pagination, hope it will help:-
VisualForce Page:-
<apex:page controller=”Pagination” sidebar=”false” showHeader=”false”>
<apex:form >
<apex:pageBlock id=”details”>
<apex:pageblockTable value=”{!acclist}” var=”acc”>
<apex:column value=”{!acc.Name}”/>
<apex:column value=”{!acc.website}”/>
<apex:column value=”{!acc.AnnualRevenue}”/>
<apex:column value=”{!acc.Description}”/>
<apex:column value=”{!acc.Type}”/>
</apex:pageblockTable>
<apex:pageblockButtons >
<apex:commandButton value=”First Page” rerender=”details” action=”{!FirstPage}” disabled=”{!prev}”/>
<apex:commandButton value=”Previous” rerender=”details” action=”{!previous}” disabled=”{!prev}”/>
<apex:commandButton value=”Next” rerender=”details” action=”{!next}” disabled=”{!nxt}”/>
<apex:commandButton value=”Last Page” rerender=”details” action=”{!LastPage}” disabled=”{!nxt}”/>
</apex:pageblockButtons>
</apex:pageBlock>
</apex:form></apex:page>Apex Controller:-
public class Pagination
{
private integer totalRecs = 0;
private integer OffsetSize = 0;
private integer LimitSize= 10;
public Pagination()
{
totalRecs = [select count() from account];
}
public List<account> getacclist()
{
List<account> acc = Database.Query(‘SELECT Name, website, AnnualRevenue, description, Type FROM account LIMIT :LimitSize OFFSET :OffsetSize’);
System.debug(‘Values are ‘ + acc);
return acc;
}
public void FirstPage()
{
OffsetSize = 0;
}
public void previous()
{
OffsetSize = OffsetSize – LimitSize;
}public void next()
{
OffsetSize = OffsetSize + LimitSize;
}public void LastPage()
{
OffsetSize = totalrecs – math.mod(totalRecs,LimitSize);
}
public boolean getprev()
{
if(OffsetSize == 0)
return true;
else
return false;
}
public boolean getnxt()
{
if((OffsetSize + LimitSize) > totalRecs)
return true;
else
return false;
}
} -
Sourabh
MemberApril 14, 2016 at 6:44 am in reply to: Can we create bar chart in lightning component?Yes sure
There is an app (Chart.js) on AppExchange. Install it in your environment and you are ready to create charts in lightning component. Link for App is provided:-
(https://appexchange.salesforce.com/listingDetail?listingId=a0N30000000q5ADEAY)
-
Sourabh
MemberApril 14, 2016 at 6:39 am in reply to: Calculation on Visualforce Page without using ControllerHi Gaurav,
Another method to calculate total of your field is by using <apex:variable>.
For example i am displaying my field in <apex:repeat>. Then i will use apex variable like this:-
<apex:variable var="i" value="{!0.00}"/>
<Table>
<apex:repeat value="{!Value}" var="item">
<tr>
<td> {!item.FieldName}
<apex:variable var="i" value="{!i+item.FieldName}"/>
</td>
</tr><tr>
<td>
Total : {!i}
</td>
</apex:repeat>
</Table>This way the total of field value will be displayed.
-
Hi Shalini,
Giving you an example of DataList:-
<apex:page >
<label>Choose a browser from this list:
<input list="browsers" name="myBrowser" /></label>
<datalist id="browsers">
<option value="Chrome"/>
<option value="Firefox"/>
<option value="Internet Explorer"/>
<option value="Opera"/>
<option value="Safari"/>
<option value="Microsoft Edge"/>
</datalist>
</apex:page>If it still not working in your Mozilla than update the version of firefox, Older versions firefox doesn't support HTML5 Datalist .
-
Sourabh
MemberApril 13, 2016 at 4:11 am in reply to: How to select all checkboxes of wrapper list on vf page using jquery?Console shows "missing attr" only if you have not included jquery. The .prop() method gets the property value for only the first element in the matched set. You can use .prop() as a replacement of .attr() in your case.